写一个C语言的7z解压缩接口
时间: 2023-07-30 14:08:34 浏览: 238
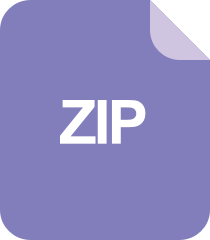
C语言编程使用lzma SDK对7z文件简单解压缩

以下是一个基本的 C 语言 7z 解压缩接口的示例代码,它使用了 7-Zip C SDK 提供的 API:
```
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <7z.h>
#include <7zCrc.h>
#include <7zFile.h>
#define kInputBufSize ((size_t)1 << 18)
int extract7zFile(const char *inFilePath, const char *outDirPath) {
int res = 0;
HANDLE hFile = NULL;
int open_res = 0;
CSzArEx db;
ISzAlloc allocImp;
ISzAlloc allocTempImp;
size_t len;
CrcGenerateTable();
allocImp.Alloc = SzAlloc;
allocImp.Free = SzFree;
allocTempImp.Alloc = SzAllocTemp;
allocTempImp.Free = SzFreeTemp;
if (InFile_Open(&hFile, inFilePath) != 0) {
return -1;
}
SzArEx_Init(&db);
open_res = SzArEx_Open(&db, hFile, &allocImp, &allocTempImp);
if (open_res == SZ_OK) {
UInt32 i;
size_t blockIndex = 0xFFFFFFFF; /* it can have any value before first call (if outBuffer = 0) */
Byte *outBuffer = 0; /* it must be 0 before first call for each new archive. */
size_t outBufferSize = 0; /* it can have any value before first call (if outBuffer = 0) */
UInt32 numErrors = 0;
for (i = 0; i < db.NumFiles; i++) {
size_t offset = 0;
size_t outSizeProcessed = 0;
size_t outSize = 0;
CSzFile outFile;
size_t len;
int isDir = SzArEx_IsDir(&db, i);
len = SzArEx_GetFileNameUtf16(&db, i, NULL);
if (len > 0) {
int *temp = (int *)malloc(len);
SzArEx_GetFileNameUtf16(&db, i, temp);
// convert filename from utf-16 to utf-8
char *fileName = (char *)malloc(len * 2 + 1);
wcstombs(fileName, (wchar_t *)temp, len * 2 + 1);
if (isDir) {
char outPath[MAX_PATH];
sprintf(outPath, "%s/%s", outDirPath, fileName);
mkdir(outPath, 0777);
} else {
res = SzArEx_Extract(&db, hFile, i,
&blockIndex, &outBuffer, &outBufferSize,
&offset, &outSizeProcessed, &outSize,
&allocImp, &allocTempImp);
if (res == SZ_OK) {
char outPath[MAX_PATH];
sprintf(outPath, "%s/%s", outDirPath, fileName);
File_Construct(&outFile);
File_Open(&outFile, outPath, FILE_CREATE | FILE_WRITE);
File_Write(&outFile, outBuffer + offset, outSize);
File_Close(&outFile);
} else {
numErrors++;
}
}
free(fileName);
free(temp);
}
SzFree(NULL, outBuffer);
outBuffer = 0;
outBufferSize = 0;
}
if (numErrors == 0) {
res = 0;
} else {
res = -1;
}
} else {
res = -1;
}
SzArEx_Free(&db, &allocImp);
InFile_Close(hFile);
return res;
}
```
上述代码中,extract7zFile() 函数接受两个参数:输入文件路径和输出目录路径。该函数首先打开输入文件,并使用 7-Zip C SDK 提供的 SzArEx_Open() 函数打开 7z 文件,并读取文件列表。
然后,对于每个文件,它检查它是否是一个目录,如果是,就在输出目录中创建一个相应的子目录。如果不是目录,就使用 SzArEx_Extract() 函数从 7z 文件中提取文件,并将其写入到输出目录中。在写入文件之前,还需要将文件名从 UTF-16 编码转换为 UTF-8 编码。
最后,该函数关闭输入文件,释放 7-Zip C SDK 分配的内存,并返回一个整数值,表示提取操作的结果。
阅读全文
相关推荐
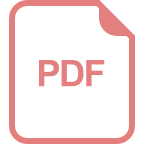
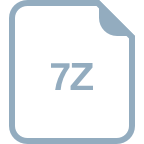
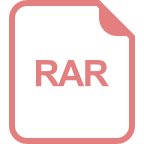
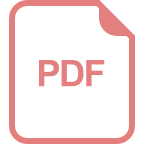
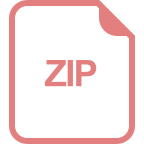
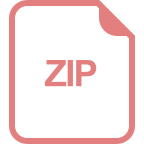
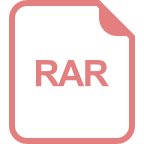
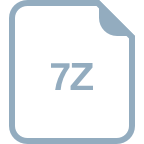
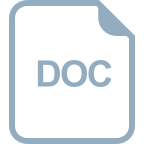
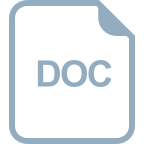
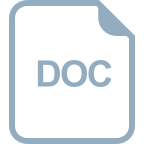
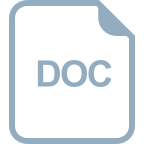
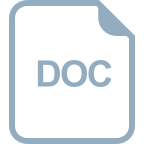
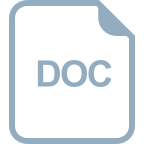
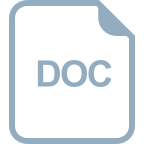
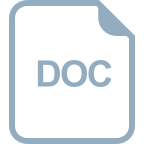
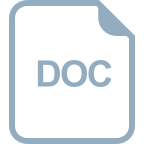