商品{storeName}{storeCode} ,可以正则取出来,反射拿对象值填充回去吗
时间: 2023-11-23 11:18:51 浏览: 92
可以的,反射可以帮助我们动态地获取对象的属性值,并将其填充到指定的字符串模板中。下面是一个示例代码:
```java
import java.lang.reflect.Field;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class ReflectDemo {
public static void main(String[] args) throws Exception {
// 假设有一个商品对象
Product product = new Product("Apple", "A001", 10.0);
// 字符串模板
String template = "商品{storeName}{storeCode}的价格为{price}元";
// 使用正则表达式匹配模板中的变量名
Pattern pattern = Pattern.compile("\\{([a-zA-Z]+)\\}");
Matcher matcher = pattern.matcher(template);
StringBuffer sb = new StringBuffer();
while (matcher.find()) {
String fieldName = matcher.group(1);
// 使用反射获取对象的属性值
Field field = product.getClass().getDeclaredField(fieldName);
field.setAccessible(true);
Object fieldValue = field.get(product);
matcher.appendReplacement(sb, fieldValue.toString());
}
matcher.appendTail(sb);
System.out.println(sb.toString()); // 输出:商品AppleA001的价格为10.0元
}
}
class Product {
private String storeName;
private String storeCode;
private double price;
public Product(String storeName, String storeCode, double price) {
this.storeName = storeName;
this.storeCode = storeCode;
this.price = price;
}
public String getStoreName() {
return storeName;
}
public void setStoreName(String storeName) {
this.storeName = storeName;
}
public String getStoreCode() {
return storeCode;
}
public void setStoreCode(String storeCode) {
this.storeCode = storeCode;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
```
在上面的代码中,我们首先定义了一个商品对象 `Product`,包含 `storeName`、`storeCode` 和 `price` 三个属性。然后我们定义了一个字符串模板 `template`,其中包含了三个变量名,即 `{storeName}`、`{storeCode}` 和 `{price}`。我们使用正则表达式匹配模板中的变量名,并使用反射获取商品对象中对应属性的值,将其填充到模板中,最终得到了一个完整的字符串。
阅读全文
相关推荐
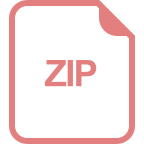
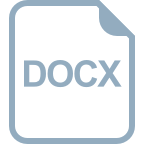
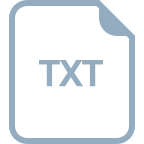















