利用Python爬取QQ音乐个人歌单,并存储在Excel文件中的代码
时间: 2024-06-10 19:06:20 浏览: 146
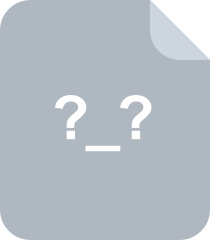
python爬虫爬取百度音乐歌单
以下是使用Python爬取QQ音乐个人歌单并存储在Excel文件中的代码:
```python
import requests
import json
import xlwt
# QQ音乐个人歌单url
url = 'https://c.y.qq.com/soso/fcgi-bin/client_search_user?p=1&n=20&w=xxx'
# 替换为自己的QQ号码
qq_number = 'xxxxxxx'
# 替换为自己的Cookie
cookie = 'xxxxxx'
# 构造请求头
headers = {
'Referer': 'https://y.qq.com/portal/profile.html',
'Cookie': cookie,
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/90.0.4430.212 Safari/537.36',
'Host': 'c.y.qq.com'
}
# 获取歌单信息
def get_playlist():
# 将QQ号码替换到url中
url = 'https://c.y.qq.com/soso/fcgi-bin/client_search_user?p=1&n=20&w='+qq_number
response = requests.get(url, headers=headers).text
json_data = json.loads(response)
playlist = []
# 获取歌单列表
for item in json_data['data']['user']['songlist']:
playlist.append({
'name': item['songname'],
'singer': item['singer'][0]['name'],
'album': item['albumname']
})
return playlist
# 将歌单数据存入Excel文件中
def save_to_excel(playlist):
# 创建Excel文件
workbook = xlwt.Workbook(encoding='utf-8')
worksheet = workbook.add_sheet('Playlist')
# 写入表头
worksheet.write(0, 0, '歌曲名')
worksheet.write(0, 1, '歌手')
worksheet.write(0, 2, '专辑')
# 写入歌单数据
row = 1
for item in playlist:
worksheet.write(row, 0, item['name'])
worksheet.write(row, 1, item['singer'])
worksheet.write(row, 2, item['album'])
row += 1
# 保存Excel文件
workbook.save('playlist.xls')
if __name__ == '__main__':
playlist = get_playlist()
save_to_excel(playlist)
```
注意事项:
1. 请将代码中的qq_number和cookie替换为你自己的相关信息。
2. 代码中的歌单信息获取方式是通过QQ号码搜索用户歌单,存在多个用户的情况下可能会出现搜索错误的情况,如遇到此情况请修改搜索关键词为个人账号的昵称。
阅读全文
相关推荐
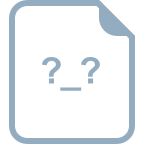
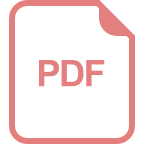
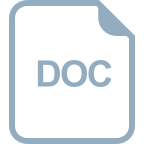
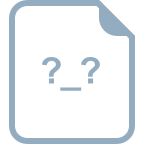
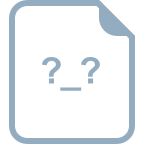
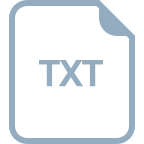
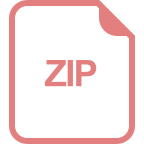
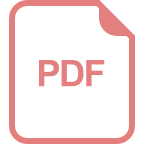
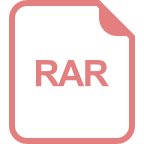
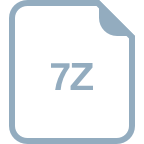
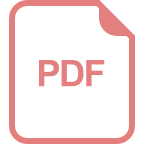
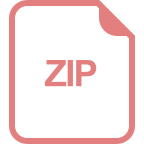
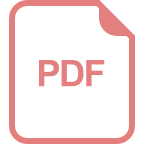
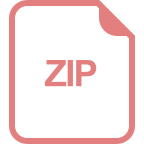