python实现将一个路径下的图片显示到控件上并且可以左右翻看
时间: 2024-10-20 14:15:17 浏览: 10
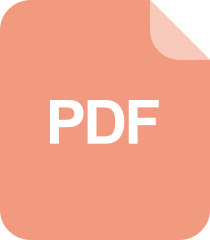
python 实现图片旋转 上下左右 180度旋转的示例

在Python中,特别是使用Tkinter库创建图形用户界面时,你可以通过以下步骤实现将图片文件显示到控件上,并提供左右切换功能:
1. 首先,你需要导入必要的库,如`os`, `PIL`(Python Imaging Library,用于图像操作)以及`tkinter`。
```python
import os
from PIL import Image, ImageTk
import tkinter as tk
```
2. 创建一个Tk窗口和一个滚动条(如果需要水平滚动),以及两个标签来显示当前和下一张图片。
```python
root = tk.Tk()
scrollbar = tk.Scrollbar(root)
img_label_left = tk.Label(root)
img_label_right = tk.Label(root)
```
3. 定义一个函数来加载并显示图片,同时处理左右切换:
```python
def load_image(path):
img = Image.open(path)
img = img.resize((400, 400)) # 可以调整尺寸
img_tk = ImageTk.PhotoImage(img)
if label_index % 2 == 0: # 判断当前标签是左侧还是右侧
img_label_left.config(image=img_tk)
img_label_left.image = img_tk
else:
img_label_right.config(image=img_tk)
img_label_right.image = img_tk
label_index += 1 # 更新图片索引
def switch_images(direction):
global label_index
path = os.path.join(directory, images[label_index])
load_image(path)
# 例如,假设`directory`变量存储了图片所在的目录,`images`是一个包含图片名称的列表
directory = "your_directory_path"
images = [f for f in os.listdir(directory) if f.endswith('.jpg')] # 获取目录下的所有.jpg文件名
label_index = 0
load_image(os.path.join(directory, images[0]))
```
4. 添加事件监听器来切换图片,比如点击按钮或者使用键盘方向键:
```python
next_button = tk.Button(root, text="Next", command=lambda: switch_images(1))
prev_button = tk.Button(root, text="Previous", command=lambda: switch_images(-1))
# 将滚动条添加到窗口,并设置其功能
scrollbar.pack(side=tk.RIGHT, fill=tk.Y)
img_label_left.pack(side=tk.LEFT, fill=tk.BOTH, expand=True)
img_label_right.pack(side=tk.RIGHT, fill=tk.BOTH, expand=True)
next_button.pack()
prev_button.pack()
root.mainloop()
```
5. 最后,记得更新`switch_images()`函数的参数值以支持滚动条或其他导航方式。
阅读全文
相关推荐
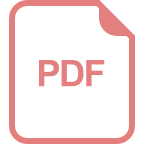
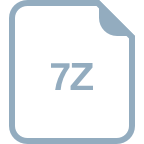
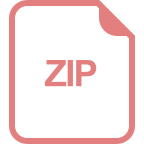
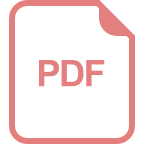
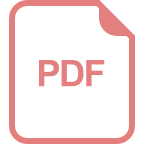
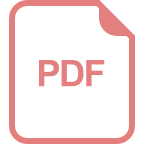
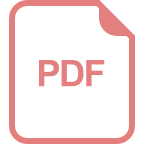
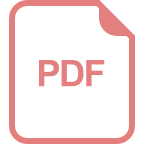
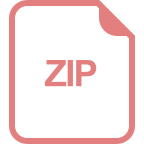
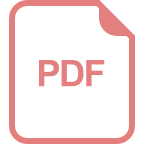
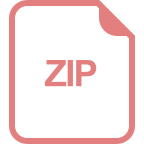
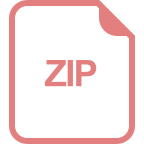
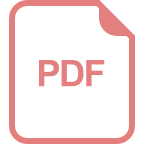
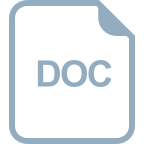