Implement a Matlab function which completes the following task: Give the best approximation of a given function f on a closed interval with respect to 2-norm (or more generally, a norm induced by an inner product).
时间: 2023-07-02 12:08:36 浏览: 79
Here is a possible implementation of the function:
```matlab
function [x, y] = best_approximation(f, a, b, n)
% Computes the best approximation of the function f on the interval [a,b]
% using n terms of the Fourier series expansion with respect to the 2-norm.
% Returns the approximation function and its values at n+1 equidistant points.
% Define the inner product on the interval [a,b]
ip = @(u, v) integral(@(x) u(x).*v(x), a, b);
% Compute the Fourier coefficients of f
a0 = 1/(b-a) * integral(f, a, b);
an = @(k) 1/(b-a) * integral(@(x) f(x).*cos(k*pi*(x-a)/(b-a)), a, b);
bn = @(k) 1/(b-a) * integral(@(x) f(x).*sin(k*pi*(x-a)/(b-a)), a, b);
% Compute the approximation function and its values
x = linspace(a, b, n+1)';
y = zeros(n+1, 1);
y(1) = a0/2;
for k = 1:n
y = y + an(k)*cos(k*pi*(x-a)/(b-a)) + bn(k)*sin(k*pi*(x-a)/(b-a));
end
```
To use this function, you need to provide a function handle to the function you want to approximate, the endpoints of the interval, and the number of terms you want to use in the Fourier series expansion. For example, to approximate the function `f(x) = x^2` on the interval `[0,1]` with 10 terms, you can call the function like this:
```matlab
f = @(x) x.^2;
a = 0;
b = 1;
n = 10;
[x, y] = best_approximation(f, a, b, n);
```
The output `x` contains the `n+1` equidistant points at which the approximation function is evaluated, and `y` contains the corresponding function values. You can plot the original function and its approximation using the following code:
```matlab
xx = linspace(a, b, 1000)';
yy = f(xx);
yyh = interp1(x, y, xx);
plot(xx, yy, 'b-', xx, yyh, 'r--');
legend('f(x)', 'approximation');
```
This will produce a plot showing the original function in blue and its approximation in red. You can adjust the number of terms in the Fourier series to see how the approximation improves with more terms.
相关推荐
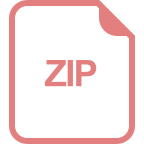
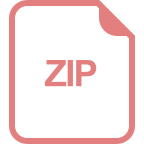
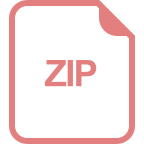















