Javaee做一个阅读记录界面的代码
时间: 2024-02-27 21:53:10 浏览: 73
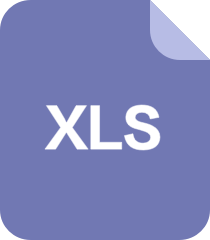
代码走读记录
以下是一个简单的JavaEE阅读记录界面代码示例,其中使用了JSP和Servlet:
1. 阅读记录界面的JSP页面(read.jsp):
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>阅读记录</title>
</head>
<body>
<h1>阅读记录</h1>
<table>
<tr>
<th>书名</th>
<th>作者</th>
<th>阅读时间</th>
<<th>操作</th>
</tr>
<c:forEach var="book" items="${books}">
<tr>
<td>${book.title}</td>
<td>${book.author}</td>
<td>${book.date}</td>
<td><a href="${pageContext.request.contextPath}/delete?id=${book.id}">删除</a></td>
</tr>
</c:forEach>
</table>
<br>
<form action="${pageContext.request.contextPath}/add" method="post">
书名:<input type="text" name="title"><br>
作者:<input type="text" name="author"><br>
阅读时间:<input type="date" name="date"><br>
<input type="submit" value="添加">
</form>
</body>
</html>
```
2. Servlet控制器(BookServlet.java):
```java
package com.example.controller;
import java.io.IOException;
import java.sql.SQLException;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.example.dao.BookDao;
import com.example.model.Book;
@WebServlet("/read")
public class BookServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private BookDao bookDao;
public BookServlet() {
super();
bookDao = new BookDao();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
List<Book> books;
try {
books = bookDao.getAllBooks();
request.setAttribute("books", books);
request.getRequestDispatcher("/read.jsp").forward(request, response);
} catch (SQLException e) {
e.printStackTrace();
response.getWriter().append("Error: " + e.getMessage());
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String title = request.getParameter("title");
String author = request.getParameter("author");
String date = request.getParameter("date");
Book book = new Book(title, author, date);
try {
bookDao.addBook(book);
response.sendRedirect(request.getContextPath() + "/read");
} catch (SQLException e) {
e.printStackTrace();
response.getWriter().append("Error: " + e.getMessage());
}
}
protected void doDelete(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
try {
bookDao.deleteBook(id);
response.sendRedirect(request.getContextPath() + "/read");
} catch (SQLException e) {
e.printStackTrace();
response.getWriter().append("Error: " + e.getMessage());
}
}
}
```
3. DAO类(BookDao.java):
```java
package com.example.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import com.example.model.Book;
import com.example.utils.DbUtils;
public class BookDao {
private Connection conn;
public BookDao() {
conn = DbUtils.getConnection();
}
public List<Book> getAllBooks() throws SQLException {
List<Book> books = new ArrayList<>();
String sql = "SELECT * FROM books";
PreparedStatement stmt = conn.prepareStatement(sql);
ResultSet rs = stmt.executeQuery();
while (rs.next()) {
int id = rs.getInt("id");
String title = rs.getString("title");
String author = rs.getString("author");
String date = rs.getString("date");
Book book = new Book(id, title, author, date);
books.add(book);
}
rs.close();
stmt.close();
return books;
}
public void addBook(Book book) throws SQLException {
String sql = "INSERT INTO books (title, author, date) VALUES (?, ?, ?)";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setString(1, book.getTitle());
stmt.setString(2, book.getAuthor());
stmt.setString(3, book.getDate());
stmt.executeUpdate();
stmt.close();
}
public void deleteBook(int id) throws SQLException {
String sql = "DELETE FROM books WHERE id=?";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setInt(1, id);
stmt.executeUpdate();
stmt.close();
}
}
```
4. 实体类(Book.java):
```java
package com.example.model;
public class Book {
private int id;
private String title;
private String author;
private String date;
public Book() {
}
public Book(String title, String author, String date) {
this.title = title;
this.author = author;
this.date = date;
}
public Book(int id, String title, String author, String date) {
this.id = id;
this.title = title;
this.author = author;
this.date = date;
}
// getters and setters
}
```
以上代码仅供参考,具体实现可能会因为需求不同而有所变化。
阅读全文
相关推荐
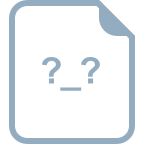
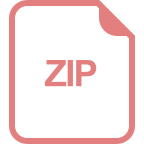
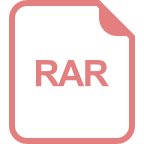
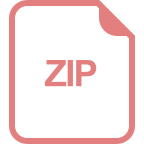
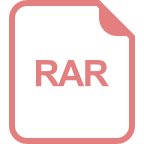
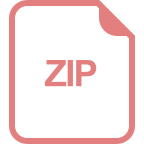
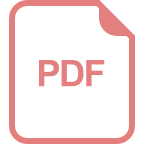
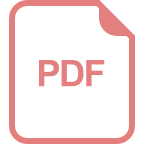
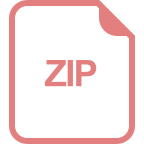
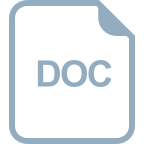
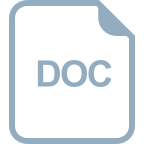
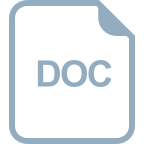
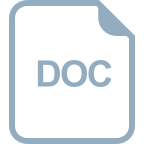
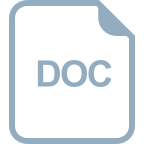
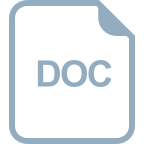
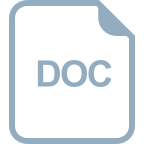

