用python语言实现:网络包括24个顶点、76条边,各条边的编号、尾节点、头节点、长度、容量已在excel表中给出,请计算所有顶点对之间的最短路长度,并绘制该网络的有向图。
时间: 2023-12-27 19:05:22 浏览: 109
好的,这是一个比较复杂的问题,需要用到图论算法和绘图库。我来逐步解释实现步骤:
1. 首先需要读取excel表格中的边信息,可以使用 pandas 库读取表格数据,具体代码如下:
```python
import pandas as pd
df = pd.read_excel('net.xlsx', sheet_name='edges')
edges = []
for i in range(len(df)):
edge = {
'id': df.loc[i, 'id'],
'tail': df.loc[i, 'tail'],
'head': df.loc[i, 'head'],
'length': df.loc[i, 'length'],
'capacity': df.loc[i, 'capacity']
}
edges.append(edge)
```
2. 接着,需要构建有向图并计算所有顶点对之间的最短路长度。这里我们可以使用 Dijkstra 算法,可以使用 networkx 库实现。具体代码如下:
```python
import networkx as nx
G = nx.DiGraph()
for edge in edges:
G.add_edge(edge['tail'], edge['head'], weight=edge['length'])
all_pairs = dict(nx.all_pairs_dijkstra_path_length(G))
```
3. 最后,需要绘制该网络的有向图。可以使用 matplotlib 库实现。具体代码如下:
```python
import matplotlib.pyplot as plt
pos = nx.spring_layout(G)
nx.draw(G, pos, with_labels=True)
labels = nx.get_edge_attributes(G, 'length')
nx.draw_networkx_edge_labels(G, pos, edge_labels=labels)
plt.show()
```
综合起来,完整的代码如下:
```python
import pandas as pd
import networkx as nx
import matplotlib.pyplot as plt
df = pd.read_excel('net.xlsx', sheet_name='edges')
edges = []
for i in range(len(df)):
edge = {
'id': df.loc[i, 'id'],
'tail': df.loc[i, 'tail'],
'head': df.loc[i, 'head'],
'length': df.loc[i, 'length'],
'capacity': df.loc[i, 'capacity']
}
edges.append(edge)
G = nx.DiGraph()
for edge in edges:
G.add_edge(edge['tail'], edge['head'], weight=edge['length'])
all_pairs = dict(nx.all_pairs_dijkstra_path_length(G))
pos = nx.spring_layout(G)
nx.draw(G, pos, with_labels=True)
labels = nx.get_edge_attributes(G, 'length')
nx.draw_networkx_edge_labels(G, pos, edge_labels=labels)
plt.show()
```
注意:这里的 excel 表格和代码中的文件名需要根据实际情况修改。
阅读全文
相关推荐
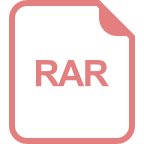




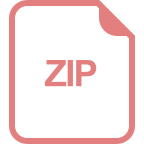
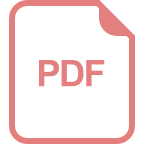
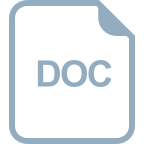
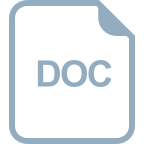







