springboot vue前后端分离项目中轮播图
时间: 2023-08-31 20:10:01 浏览: 686
在Spring Boot和Vue前后端分离项目中实现轮播图,可以考虑以下步骤:
1. 在后端Spring Boot项目中创建一个Controller,提供获取轮播图数据的接口。
2. 在后端项目中定义一个Model类,用于存储轮播图数据。
3. 使用Spring Data JPA或其他ORM框架,将轮播图数据保存到数据库中。
4. 在前端Vue项目中创建一个轮播图组件,并通过调用后端提供的接口,获取轮播图数据。
5. 使用Vue插件(如vue-awesome-swiper)或手动实现轮播图效果。
以下是一个简单的示例:
后端Spring Boot项目中的Controller:
```java
@RestController
@RequestMapping("/api")
public class CarouselController {
@Autowired
private CarouselRepository carouselRepository;
@GetMapping("/carousel")
public List<Carousel> getCarousel() {
return carouselRepository.findAll();
}
}
```
后端Spring Boot项目中的Model类:
```java
@Entity
@Table(name = "carousel")
public class Carousel {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String imageUrl;
// getter和setter
}
```
前端Vue项目中的轮播图组件:
```vue
<template>
<div>
<swiper :options="swiperOption">
<swiper-slide v-for="item in carouselData" :key="item.id">
<img :src="item.imageUrl" />
</swiper-slide>
</swiper>
</div>
</template>
<script>
import axios from "axios";
import { Swiper, SwiperSlide } from "vue-awesome-swiper";
import "swiper/swiper-bundle.css";
export default {
data() {
return {
carouselData: [],
swiperOption: {
loop: true,
autoplay: {
delay: 3000,
disableOnInteraction: false,
},
pagination: {
el: ".swiper-pagination",
clickable: true,
},
},
};
},
components: {
Swiper,
SwiperSlide,
},
mounted() {
axios.get("/api/carousel").then((response) => {
this.carouselData = response.data;
});
},
};
</script>
```
这个示例中,我们使用了Spring Boot作为后端框架,Vue作为前端框架,并使用了vue-awesome-swiper插件实现轮播图效果。在后端,我们使用了JPA和MySQL作为ORM框架和数据库。在前端,我们通过调用后端提供的接口获取轮播图数据,并使用Swiper组件展示轮播图。
阅读全文
相关推荐
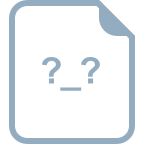
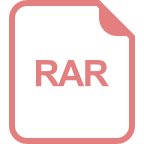
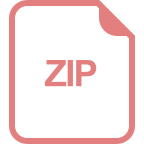
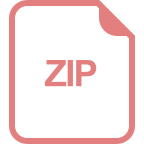
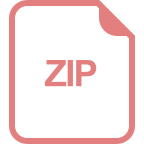
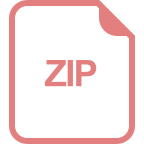
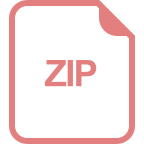
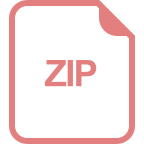
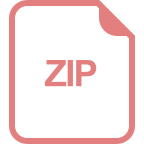
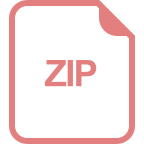
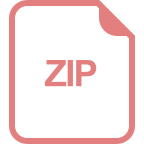
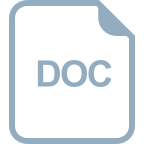
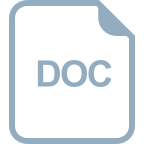
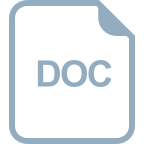
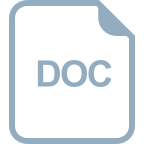
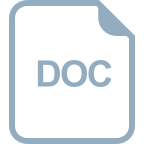
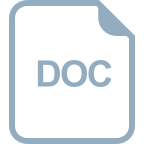