本题的目标是计算布尔表达式,例如:(V|V)&F&(F|V) 其中 V 表示 True,F 表示 False。 表达式可以包含下述运算符: ! 表示not,& 表示and,| 表示 or ,允许使用括号。 为了执行表达式的运算,要考虑运算符的优先级:not的优先级最高,or的优先级最低。程序要产生 V 或 F,即输入文件中每个表达式的结果。 输入描述 一个表达式不超过100个符号。符号间可以用任意个空格分开,也可以没有空格,所以表达式的总长度,也就是字符的个数,是未知的。 在输入中,表达式的个数是一个变量,不大于20。每个表达式在一行中,如样例所示。 输出描述 对测试用例中的每个表达式,输出Expression,后面跟着序列号和:,然后是相应测试表达式的结果值。 每个测试表达式一行。 使用如下所示的样例输出中的格式。 用例输入 1 (V|V)&F&(F|V) !V|V&V&!F&(F|V)&(!F|F|!V&V) (F&F|V|!V&!F&!(F|F&V)) 用例输出 1 Expression 1: F Expression 2: V Expression 3: V c++
时间: 2024-03-08 15:50:10 浏览: 99
以下是C++代码实现,采用了递归的方式对表达式进行求解:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <stack>
#include <algorithm>
using namespace std;
// 定义布尔值类型
enum BooleanValue { V = true, F = false };
// 递归求解布尔表达式
BooleanValue eval(const string& exp, int& idx);
// 求解逻辑非表达式
BooleanValue eval_not(const string& exp, int& idx);
// 求解逻辑与和逻辑或表达式
BooleanValue eval_and_or(const string& exp, int& idx);
// 判断字符是否是空格
bool is_space(char c) {
return c == ' ';
}
// 去除表达式中的空格
string remove_spaces(const string& exp) {
string res;
remove_copy_if(exp.begin(), exp.end(), back_inserter(res), is_space);
return res;
}
// 计算布尔表达式
BooleanValue eval_expression(const string& exp) {
int idx = 0;
return eval(exp, idx);
}
// 递归求解布尔表达式
BooleanValue eval(const string& exp, int& idx) {
if (exp[idx] == '(') {
idx++; // 跳过左括号
BooleanValue res = eval_and_or(exp, idx);
idx++; // 跳过右括号
return res;
}
else {
return eval_not(exp, idx);
}
}
// 求解逻辑非表达式
BooleanValue eval_not(const string& exp, int& idx) {
if (exp[idx] == '!') {
idx++; // 跳过逻辑非符号
BooleanValue res = eval_not(exp, idx);
return (res == V) ? F : V; // 对逻辑非表达式的结果进行取反
}
else {
return eval_and_or(exp, idx);
}
}
// 求解逻辑与和逻辑或表达式
BooleanValue eval_and_or(const string& exp, int& idx) {
stack<BooleanValue> values; // 存储值的栈
stack<char> ops; // 存储运算符的栈
// 进行循环求解
while (idx < exp.size()) {
if (exp[idx] == '(') {
idx++; // 跳过左括号
BooleanValue res = eval_and_or(exp, idx);
values.push(res);
}
else if (exp[idx] == ')') {
break; // 遇到右括号退出循环
}
else {
BooleanValue val;
if (exp[idx] == 'V') {
val = V;
}
else if (exp[idx] == 'F') {
val = F;
}
else {
throw runtime_error(string("invalid character: ") + exp[idx]);
}
if (!ops.empty() && ops.top() == '&') {
ops.pop();
BooleanValue v1 = values.top();
values.pop();
BooleanValue res = (v1 == V && val == V) ? V : F; // 计算逻辑与表达式
values.push(res);
}
else if (!ops.empty() && ops.top() == '|') {
ops.pop();
BooleanValue v1 = values.top();
values.pop();
BooleanValue res = (v1 == V || val == V) ? V : F; // 计算逻辑或表达式
values.push(res);
}
else {
values.push(val);
}
}
idx++; // 跳过当前字符
}
// 处理剩余的运算符
while (!ops.empty()) {
char op = ops.top();
ops.pop();
BooleanValue v2 = values.top();
values.pop();
BooleanValue v1 = values.top();
values.pop();
if (op == '&') {
BooleanValue res = (v1 == V && v2 == V) ? V : F; // 计算逻辑与表达式
values.push(res);
}
else if (op == '|') {
BooleanValue res = (v1 == V || v2 == V) ? V : F; // 计算逻辑或表达式
values.push(res);
}
}
return values.top(); // 返回表达式的值
}
int main() {
int n;
cin >> n;
cin.ignore(); // 忽略换行符
for (int i = 1; i <= n; ++i) {
string exp;
getline(cin, exp); // 读取表达式
exp = remove_spaces(exp); // 去除表达式中的空格
BooleanValue res = eval_expression(exp); // 计算表达式的值
cout << "Expression " << i << ": " << (res == V ? "V" : "F") << endl; // 输出结果
}
return 0;
}
```
阅读全文
相关推荐
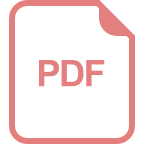
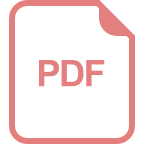
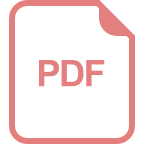

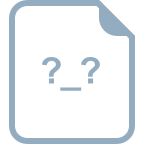
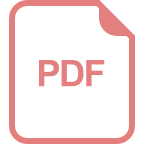
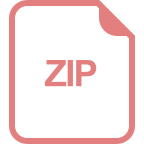
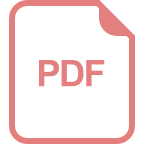
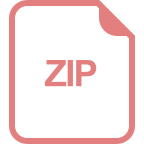
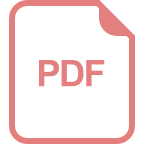
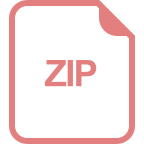
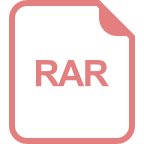
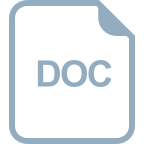
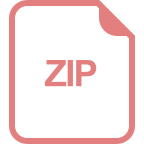
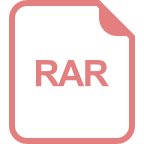
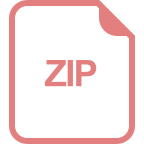
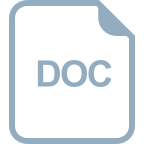
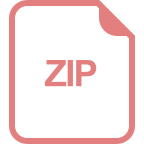
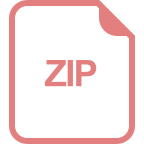