要求实现 页面1: 登录页面,有两个输入框,分别输入用户名,密码。 有两个按钮,登录、注册。 点击登录按钮,用输入的用户名、密码和数据库中的用户名密码匹配,正确则进入页面3,错误则给出提示。 点击注册按钮,进入页面2 页面2: 输入用户信息,包括、用户名、密码、姓名、学号、班级、电话。 有一个提交按钮,点击提交后,把数据存入数据库,并跳转页面1. 页面3: 显示数据库中所有用户信息。包括: 姓名、学号、班级、电话。 在每行列表后,加入添加、删除功能。 点击添加:进入页面2,并将相关信息显示在输入框中。 点击删除:删除本行,并刷新页面3,显示当前列表内容。 设置退出功能,点击退出返回页面1用php代码实现其中数据库的登录用户为root 密码为roo 表名users
时间: 2024-02-09 18:10:23 浏览: 21
好的,这是一个比较完整的网站功能,需要用到 HTML、CSS、JavaScript 和 PHP。首先我们需要在服务器上创建一个名为 "users" 的数据库,其中包含一个名为 "user_info" 的表,表中包含以下字段:id、username、password、name、student_id、class、phone。
接下来,我们可以按照题目要求,分别实现页面1、页面2、页面3:
页面1:登录页面
```
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
</head>
<body>
<form method="post" action="login.php">
<input type="text" name="username" placeholder="Username"><br>
<input type="password" name="password" placeholder="Password"><br>
<button type="submit" name="login">Login</button>
<button type="button" onclick="location.href='register.php'">Register</button>
</form>
</body>
</html>
```
在上面的代码中,我们创建了一个表单,包含两个输入框和两个按钮,分别用于输入用户名、密码,以及登录和注册。
页面2:注册页面
```
<!DOCTYPE html>
<html>
<head>
<title>Register</title>
</head>
<body>
<form method="post" action="register.php">
<input type="text" name="username" placeholder="Username"><br>
<input type="password" name="password" placeholder="Password"><br>
<input type="text" name="name" placeholder="Name"><br>
<input type="text" name="student_id" placeholder="Student ID"><br>
<input type="text" name="class" placeholder="Class"><br>
<input type="text" name="phone" placeholder="Phone"><br>
<button type="submit" name="register">Register</button>
</form>
</body>
</html>
```
在上面的代码中,我们创建了一个表单,包含多个输入框和一个提交按钮,用于输入用户信息并提交到服务器。
页面3:用户信息页面
```
<!DOCTYPE html>
<html>
<head>
<title>User Info</title>
<script type="text/javascript">
function deleteUser(id) {
if (confirm("Are you sure to delete this user?")) {
location.href = "delete.php?id=" + id;
}
}
</script>
</head>
<body>
<table>
<tr>
<th>Name</th>
<th>Student ID</th>
<th>Class</th>
<th>Phone</th>
<th>Action</th>
</tr>
<?php
$conn = mysqli_connect("localhost", "root", "root", "users");
$query = "SELECT * FROM user_info";
$result = mysqli_query($conn, $query);
while ($row = mysqli_fetch_assoc($result)) {
echo "<tr>";
echo "<td>" . $row["name"] . "</td>";
echo "<td>" . $row["student_id"] . "</td>";
echo "<td>" . $row["class"] . "</td>";
echo "<td>" . $row["phone"] . "</td>";
echo "<td><button type='button' onclick='deleteUser(" . $row["id"] . ")'>Delete</button></td>";
echo "</tr>";
}
mysqli_close($conn);
?>
</table>
<button type="button" onclick="location.href='register.php'">Add</button>
<button type="button" onclick="location.href='logout.php'">Logout</button>
</body>
</html>
```
在上面的代码中,我们创建了一个表格,用于显示数据库中所有用户信息,并为每行列表添加了删除功能。同时,我们还添加了一个 "Add" 按钮用于添加新用户,以及一个 "Logout" 按钮用于退出登录。
除此之外,我们还需要编写相应的 PHP 脚本,用于处理登录、注册、删除和退出操作。下面是大致的代码:
login.php
```
<?php
session_start();
$conn = mysqli_connect("localhost", "root", "root", "users");
$username = $_POST["username"];
$password = $_POST["password"];
$query = "SELECT * FROM user_info WHERE username='$username' AND password='$password'";
$result = mysqli_query($conn, $query);
if (mysqli_num_rows($result) == 1) {
$_SESSION["username"] = $username;
header("Location: user_info.php");
} else {
echo "Invalid username or password.";
}
mysqli_close($conn);
?>
```
register.php
```
<?php
$conn = mysqli_connect("localhost", "root", "root", "users");
$username = $_POST["username"];
$password = $_POST["password"];
$name = $_POST["name"];
$student_id = $_POST["student_id"];
$class = $_POST["class"];
$phone = $_POST["phone"];
$query = "INSERT INTO user_info (username, password, name, student_id, class, phone) VALUES ('$username', '$password', '$name', '$student_id', '$class', '$phone')";
mysqli_query($conn, $query);
mysqli_close($conn);
header("Location: login.php");
?>
```
delete.php
```
<?php
$conn = mysqli_connect("localhost", "root", "root", "users");
$id = $_GET["id"];
$query = "DELETE FROM user_info WHERE id=$id";
mysqli_query($conn, $query);
mysqli_close($conn);
header("Location: user_info.php");
?>
```
logout.php
```
<?php
session_start();
session_destroy();
header("Location: login.php");
?>
```
至此,我们已经完成了一个简单的用户管理系统。当然,这只是一个初步的实现,还有很多细节可以进一步完善,如表单验证、密码加密、安全性等等。
相关推荐
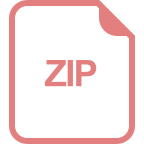
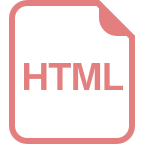
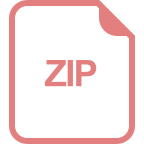
















