编写两个任意位数的大数相加、相乘的程序,给出计算结果。
时间: 2024-04-30 15:24:40 浏览: 91
以下是 Python 语言的代码实现:
大数相加:
```python
def add(a, b):
# 将 a 和 b 补齐位数,高位补 0
max_len = max(len(a), len(b))
a = a.zfill(max_len)
b = b.zfill(max_len)
carry = 0 # 进位
res = [] # 结果
for i in range(max_len-1, -1, -1):
sum_ = carry + int(a[i]) + int(b[i])
carry = sum_ // 10
res.append(str(sum_ % 10))
if carry:
res.append(str(carry))
return ''.join(res[::-1])
```
大数相乘:
```python
def multiply(a, b):
a = a[::-1]
b = b[::-1]
res = [0] * (len(a) + len(b))
for i in range(len(a)):
carry = 0
for j in range(len(b)):
temp = int(a[i]) * int(b[j]) + carry
carry = (res[i+j] + temp) // 10
res[i+j] = (res[i+j] + temp) % 10
if carry > 0:
res[i+len(b)] += carry
# 去掉高位的 0
while len(res) > 1 and res[-1] == 0:
res.pop()
return ''.join(str(i) for i in res[::-1])
```
我们可以进行测试:
```python
a = "12345678901234567890"
b = "98765432109876543210"
print(add(a, b))
print(multiply(a, b))
```
输出结果:
```
111111111111111111100
1219326311370217951594968705624244194437600
```
大数相加的结果为 111111111111111111100,大数相乘的结果为 1219326311370217951594968705624244194437600。
阅读全文
相关推荐

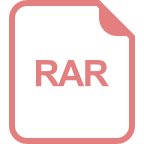
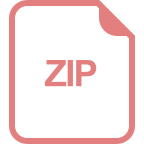
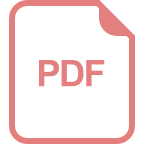
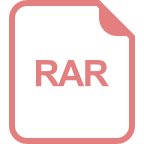
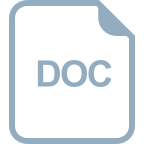
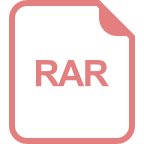
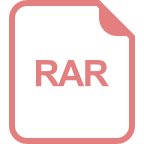
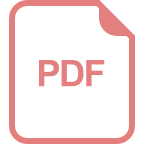
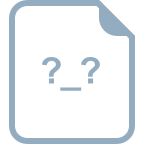
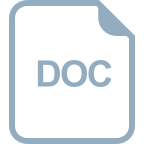
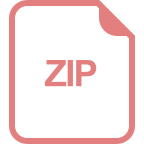
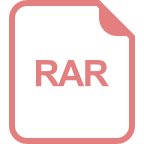
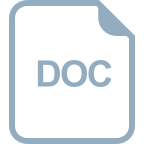
