for b in bullets: if b.active: b.move() screen.blit(b.image, b.rect) enemy_hit = pygame.sprite.spritecollide(b, enemies, False, pygame.sprite.collide_mask) if enemy_hit: b.active = False for e in enemy_hit: if e in mid_enemies or e in big_enemies: e.hit = True e.energy -= 1 if e.energy == 0: e.active = False else: e.active = False
时间: 2023-06-17 22:07:01 浏览: 176
这段代码看起来是一个游戏中的子弹和敌人的碰撞检测部分。
首先,遍历所有的子弹列表。如果子弹处于激活状态,就让它移动,并将它的图像绘制在屏幕上。
随后,使用 `pygame.sprite.spritecollide()` 函数检测子弹是否与敌人发生了碰撞。如果发生了碰撞,就将子弹的活动状态设置为不活动,然后遍历所有与子弹发生碰撞的敌人。如果敌人是中等或大型敌人,就将它的 `hit` 标记设置为 `True`,并将它的能量值减 1。如果敌人的能量值降到了 0,就将它的活动状态设置为不活动。如果敌人是小型敌人,就直接将它的活动状态设置为不活动。
相关问题
def __check_cash(self): """碰撞检测""" # 1. 检测子弹与敌机 start_num = len(self.hero.bullets) for enemy in self.enemy_group: if enemy.modnum == 1: if pygame.sprite.spritecollide(enemy, self.hero.bullets, True): self.hero.bullets_num += 2 enemy.modnum = 2 end_num = len(self.hero.bullets) self.scores += start_num - end_num # 2. 检测敌机与英雄 enemies = pygame.sprite.spritecollide(self.hero, self.enemy_group, False) if enemies: for n in (1, 2, 3, 4): time.sleep(0.2) self.screen.blit(pygame.image.load("./images/me_destroy_%d.png" % n), self.hero.rect) self.screen.blit(pygame.image.load("./images/enemy1_down%d.png" % n), enemies[0].rect) pygame.display.update() self.hero.kill() self.__game_over() 解释一下
这段代码是一个游戏中的碰撞检测函数,在游戏中主要负责检测子弹与敌机、敌机与英雄之间的碰撞。函数中包含了两个部分的碰撞检测。
第一部分的碰撞检测是检测子弹与敌机之间的碰撞。首先,我们使用了`len()`函数获取了英雄飞机中当前子弹的数量,然后对于每个敌机,我们使用`pygame.sprite.spritecollide()`方法检测它是否与英雄飞机的子弹相撞。如果发生碰撞,我们会将子弹销毁,并将敌机的modnum属性设为2,以标记该敌机已被击中。同时,我们还会根据销毁的子弹数量计算得分。
第二部分的碰撞检测是检测敌机与英雄飞机之间的碰撞。我们使用了`pygame.sprite.spritecollide()`方法检测是否有敌机与英雄飞机相撞,如果存在碰撞,我们会播放英雄飞机爆炸和敌机爆炸的动画,然后将英雄飞机删除并结束游戏。
值得注意的是,为了在播放爆炸动画时使其更加流畅,我们使用了`time.sleep()`方法来暂停程序的执行,以便让动画能够展现出来。同时,在播放完爆炸动画后,我们会调用`pygame.display.update()`方法来更新屏幕,以让用户能够看到爆炸动画的效果。
总之,这段代码的主要作用是实现游戏中的碰撞检测功能,以便让游戏能够更加流畅地进行。
利用pygame模块实现飞机大战附加功能如下: (1) 实现碰撞检测(可以消灭敌机,也可以被敌机消灭) (2) 随机出现云朵在天空中漂浮 (3) 随机出现甜甜圈进行能量补给(生命力或子弹类型发生变化) (4) 有GAME OVER条件控制处理
好的,以下是我的实现思路和代码示例:
1. 碰撞检测:首先需要定义玩家飞机和敌机的矩形区域,然后使用pygame自带的colliderect()函数检测两个矩形是否相交,如果相交则代表碰撞发生,可以进行相应的处理(比如减少生命值或消灭敌机)。同理,也可以检测子弹和敌机之间的碰撞。
2. 云朵:可以在游戏初始化时生成一些云朵的坐标,然后在游戏循环中不断地更新云朵的位置,让它们看起来像是在天空中漂浮。同时也可以给云朵添加一些随机的动画效果,比如缓慢移动或逐渐消失等。
3. 能量补给:同样可以在游戏初始化时生成一些甜甜圈的坐标,然后在游戏循环中检测玩家飞机和甜甜圈之间的碰撞,如果碰撞发生则可以根据甜甜圈的类型增加生命值或子弹类型等。
4. GAME OVER:可以设置一些游戏结束的条件,比如生命值降到0或者时间超过一定限制等。当满足这些条件时,就可以结束游戏并显示相应的游戏结束画面。
以下是一份简单的示例代码,仅供参考:
```python
import pygame
import random
# 初始化游戏
pygame.init()
screen = pygame.display.set_mode((480, 700))
pygame.display.set_caption("飞机大战")
clock = pygame.time.Clock()
# 加载资源
bg_img = pygame.image.load("images/background.png").convert()
player_img = pygame.image.load("images/me1.png").convert_alpha()
enemy_imgs = [pygame.image.load("images/e1.png").convert_alpha(),
pygame.image.load("images/e2.png").convert_alpha(),
pygame.image.load("images/e3.png").convert_alpha(),
pygame.image.load("images/e4.png").convert_alpha()]
bullet_imgs = [pygame.image.load("images/bullet1.png").convert_alpha(),
pygame.image.load("images/bullet2.png").convert_alpha()]
cloud_img = pygame.image.load("images/cloud.png").convert_alpha()
supply_imgs = [pygame.image.load("images/bomb_supply.png").convert_alpha(),
pygame.image.load("images/bullet_supply.png").convert_alpha()]
# 定义游戏对象
class GameObject(pygame.sprite.Sprite):
def __init__(self, img, pos):
pygame.sprite.Sprite.__init__(self)
self.image = img
self.rect = self.image.get_rect()
self.rect.left, self.rect.top = pos
class Player(GameObject):
def __init__(self, img, pos):
super().__init__(img, pos)
self.speed = 5
self.bullets = pygame.sprite.Group()
self.life = 3
def move_up(self):
self.rect.top -= self.speed
if self.rect.top < 0:
self.rect.top = 0
def move_down(self):
self.rect.top += self.speed
if self.rect.bottom > 700:
self.rect.bottom = 700
def move_left(self):
self.rect.left -= self.speed
if self.rect.left < 0:
self.rect.left = 0
def move_right(self):
self.rect.left += self.speed
if self.rect.right > 480:
self.rect.right = 480
def fire(self):
bullet = Bullet(bullet_imgs[0], (self.rect.centerx - 5, self.rect.top - 20))
self.bullets.add(bullet)
class Enemy(GameObject):
def __init__(self, img, pos):
super().__init__(img, pos)
self.speed = random.randint(2, 4)
self.life = 1
def move(self):
self.rect.top += self.speed
class Bullet(GameObject):
def __init__(self, img, pos):
super().__init__(img, pos)
self.speed = 8
def move(self):
self.rect.top -= self.speed
class Cloud(GameObject):
def __init__(self, img, pos):
super().__init__(img, pos)
self.speed = random.randint(1, 3)
def move(self):
self.rect.left += self.speed
class Supply(GameObject):
def __init__(self, img, pos, kind):
super().__init__(img, pos)
self.speed = 5
self.kind = kind
def move(self):
self.rect.top += self.speed
# 创建游戏对象实例
player = Player(player_img, (240, 600))
enemies = pygame.sprite.Group()
bullets = pygame.sprite.Group()
clouds = pygame.sprite.Group()
supplies = pygame.sprite.Group()
# 定义游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
player.move_up()
elif event.key == pygame.K_DOWN:
player.move_down()
elif event.key == pygame.K_LEFT:
player.move_left()
elif event.key == pygame.K_RIGHT:
player.move_right()
elif event.key == pygame.K_SPACE:
player.fire()
# 生成敌机
if random.randint(1, 80) == 1:
enemy_img = random.choice(enemy_imgs)
enemy_pos = (random.randint(0, 480 - enemy_img.get_width()), -enemy_img.get_height())
enemy = Enemy(enemy_img, enemy_pos)
enemies.add(enemy)
# 生成云朵
if random.randint(1, 60) == 1:
cloud_pos = (random.randint(0, 480 - cloud_img.get_width()), -cloud_img.get_height())
cloud = Cloud(cloud_img, cloud_pos)
clouds.add(cloud)
# 生成能量补给
if random.randint(1, 300) == 1:
supply_kind = random.randint(0, 1)
supply_img = supply_imgs[supply_kind]
supply_pos = (random.randint(0, 480 - supply_img.get_width()), -supply_img.get_height())
supply = Supply(supply_img, supply_pos, supply_kind)
supplies.add(supply)
# 更新游戏对象的位置
for enemy in enemies:
enemy.move()
for bullet in bullets:
bullet.move()
for cloud in clouds:
cloud.move()
for supply in supplies:
supply.move()
# 检测碰撞
for enemy in enemies:
if pygame.sprite.collide_rect(enemy, player):
player.life -= 1
enemies.remove(enemy)
else:
for bullet in bullets:
if pygame.sprite.collide_rect(enemy, bullet):
enemy.life -= 1
bullets.remove(bullet)
if enemy.life <= 0:
enemies.remove(enemy)
for supply in supplies:
if pygame.sprite.collide_rect(supply, player):
if supply.kind == 0:
player.life += 1
else:
bullet_imgs.reverse()
supplies.remove(supply)
# 绘制游戏界面
screen.blit(bg_img, (0, 0))
screen.blit(player.image, player.rect)
for enemy in enemies:
screen.blit(enemy.image, enemy.rect)
for bullet in bullets:
screen.blit(bullet.image, bullet.rect)
for cloud in clouds:
screen.blit(cloud.image, cloud.rect)
for supply in supplies:
screen.blit(supply.image, supply.rect)
# 绘制生命值和子弹类型
font = pygame.font.Font(None, 24)
life_text = font.render("Life: " + str(player.life), True, (255, 0, 0))
bullet_text = font.render("Bullet Type: " + ("Normal" if bullet_imgs[0] == bullet_imgs[-1] else "Special"), True, (255, 0, 0))
screen.blit(life_text, (10, 10))
screen.blit(bullet_text, (10, 30))
# 处理游戏结束条件
if player.life <= 0:
gameover_img = pygame.image.load("images/gameover.png").convert_alpha()
screen.blit(gameover_img, ((480 - gameover_img.get_width()) // 2, (700 - gameover_img.get_height()) // 2))
pygame.display.update()
pygame.time.wait(3000)
pygame.quit()
exit()
# 更新屏幕
pygame.display.update()
clock.tick(60)
```
以上代码仅实现了部分功能,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
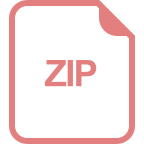
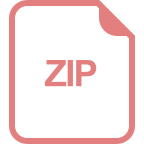
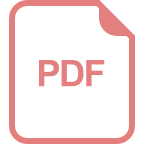












