java怎么实现把本地文件通过跳板机登录到服务器1中的然后在重服务器1把本地文件通过传输到服务器二
时间: 2024-06-08 22:07:34 浏览: 83
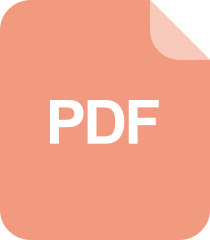
Java上传文件到服务器端的方法

实现这个功能,可以采用以下步骤:
1. 通过Java程序连接跳板机,可以使用JSch库实现。具体可以参考 https://www.jianshu.com/p/2dcb5a5d6bcb 中的代码。
2. 连接成功后,使用JSch的端口转发功能,在本地建立一个端口,使得通过本地端口可以访问服务器1。
3. 在本地读取文件,使用FTP或者SFTP等协议将文件上传到服务器1上。
4. 在服务器1上执行命令,将文件从服务器1上传到服务器2上。可以使用SCP命令实现,例如:
```
scp /path/to/local/file user@server2:/path/to/remote/directory
```
这个命令会将本地文件上传到服务器2上的指定目录下。
完整的代码实现可以参考以下示例:
```java
import com.jcraft.jsch.*;
import java.io.*;
import java.util.Properties;
public class FileTransfer {
private static final String JUMP_SERVER_HOST = "jump.server.host";
private static final String JUMP_SERVER_USERNAME = "jump.server.username";
private static final String JUMP_SERVER_PASSWORD = "jump.server.password";
private static final String SERVER1_HOST = "server1.host";
private static final String SERVER1_USERNAME = "server1.username";
private static final String SERVER2_HOST = "server2.host";
private static final String SERVER2_USERNAME = "server2.username";
public static void main(String[] args) {
String localFilePath = "/path/to/local/file";
String remoteDirectory = "/path/to/remote/directory";
// Connect to jump server
JSch jsch = new JSch();
String jumpServerHost = System.getProperty(JUMP_SERVER_HOST);
String jumpServerUsername = System.getProperty(JUMP_SERVER_USERNAME);
String jumpServerPassword = System.getProperty(JUMP_SERVER_PASSWORD);
Session jumpSession;
try {
jumpSession = jsch.getSession(jumpServerUsername, jumpServerHost, 22);
jumpSession.setPassword(jumpServerPassword);
Properties config = new Properties();
config.put("StrictHostKeyChecking", "no");
jumpSession.setConfig(config);
jumpSession.connect();
// Forward local port to server1
int localPort = 12345;
int server1Port = 22;
String server1Host = System.getProperty(SERVER1_HOST);
String server1Username = System.getProperty(SERVER1_USERNAME);
int assignedPort = jumpSession.setPortForwardingL(localPort, server1Host, server1Port);
// Connect to server1
JSch server1Jsch = new JSch();
Session server1Session = server1Jsch.getSession(server1Username, "127.0.0.1", assignedPort);
server1Session.setPassword(jumpServerPassword);
server1Session.setConfig(config);
server1Session.connect();
// Upload file to server1
ChannelSftp sftpChannel = (ChannelSftp) server1Session.openChannel("sftp");
sftpChannel.connect();
sftpChannel.put(new FileInputStream(localFilePath), remoteDirectory + "/" + new File(localFilePath).getName());
sftpChannel.disconnect();
// Transfer file from server1 to server2
String server2Host = System.getProperty(SERVER2_HOST);
String server2Username = System.getProperty(SERVER2_USERNAME);
String scpCommand = "scp " + remoteDirectory + "/" + new File(localFilePath).getName() + " " + server2Username + "@" + server2Host + ":" + remoteDirectory;
Channel channel = server1Session.openChannel("exec");
((ChannelExec) channel).setCommand(scpCommand);
channel.connect();
InputStream in = channel.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
channel.disconnect();
// Disconnect from server1
server1Session.disconnect();
} catch (JSchException | IOException | SftpException e) {
e.printStackTrace();
}
}
}
```
注意,上述代码中需要在命令行中传入以下参数:
```
-Djump.server.host=jump_server_host
-Djump.server.username=jump_server_username
-Djump.server.password=jump_server_password
-Dserver1.host=server1_host
-Dserver1.username=server1_username
-Dserver2.host=server2_host
-Dserver2.username=server2_username
```
其中,jump_server_host、jump_server_username、jump_server_password分别为跳板机的主机名、用户名和密码;server1_host、server1_username为目标服务器1的主机名和用户名;server2_host、server2_username为目标服务器2的主机名和用户名。
阅读全文
相关推荐
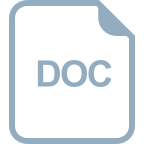
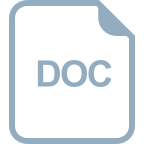



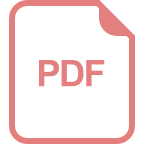
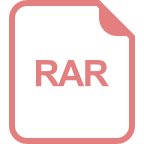
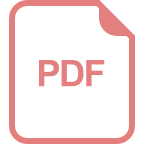
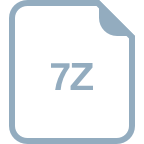
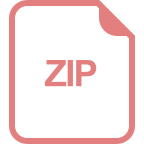
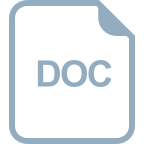
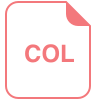
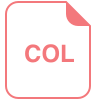
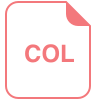
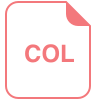
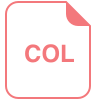
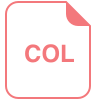
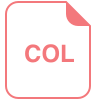