编写python程序读取文件,旅游收入.txt,将地区名称与旅游收入分别存储在两个列表中,利用matplotlib库绘制直方图
时间: 2024-03-02 20:50:58 浏览: 17
好的,以下是示例代码:
```python
import matplotlib.pyplot as plt
# 读取文件并将信息存储在两个列表中
with open('旅游收入.txt', 'r') as f:
data = f.read().splitlines()
regions = []
incomes = []
for line in data:
region, income = line.split(',')
regions.append(region)
incomes.append(int(income))
# 绘制直方图
plt.hist(incomes, bins=10, alpha=0.5, color='purple')
# 设置图表标题和坐标轴标签
plt.title('Tourism Income')
plt.xlabel('Income')
plt.ylabel('Frequency')
# 显示图表
plt.show()
```
同样的,`旅游收入.txt` 是文件名,需要根据实际情况修改。我们使用 `splitlines()` 函数读取文件内容,并将每行数据按逗号分割为两部分,分别存储在 `regions` 和 `incomes` 两个列表中。然后,用 `plt.hist()` 函数绘制直方图。请注意,这里只使用了 `incomes` 列表作为绘制直方图的数据,如果需要绘制不同地区的直方图,可以对应修改。
相关问题
编写python程序读取文件,旅游收入.txt,将地区名称与旅游收入的数据存储在两个列表中,利用matplotlib库绘制直方图
好的,我可以帮你解决这个问题。以下是Python程序的代码:
```python
import matplotlib.pyplot as plt
regions = []
incomes = []
with open('旅游收入.txt', 'r') as file:
lines = file.readlines()
for line in lines:
region, income = line.strip().split('\t')
regions.append(region)
incomes.append(int(income))
plt.bar(regions, incomes)
plt.title('Tourism Incomes')
plt.xlabel('Regions')
plt.ylabel('Incomes')
plt.show()
```
这个程序会读取文件`旅游收入.txt`,并将每行的地区名称和旅游收入数据分别存储在`regions`和`incomes`列表中。然后,使用`matplotlib`库绘制一个直方图,横轴表示地区名称,纵轴表示旅游收入。最后,使用`plt.show()`方法显示这个图表。
编写python程序读取文件:regions.txt和incomes.txt,分别存储在两个列表里,利用matplotlib库绘制直方图
好的,这是一个比较常见的数据可视化任务,我来给您提供一个 Python 代码,帮您实现这个需求。
```python
import matplotlib.pyplot as plt
# 读取文件
with open('regions.txt', 'r') as f_regions:
regions = [line.strip() for line in f_regions.readlines()]
with open('incomes.txt', 'r') as f_incomes:
incomes = [float(line.strip()) for line in f_incomes.readlines()]
# 绘制直方图
plt.bar(range(len(incomes)), incomes, tick_label=regions)
plt.title('Regional Incomes')
plt.xlabel('Regions')
plt.ylabel('Incomes')
plt.show()
```
这段代码中,我们首先使用 `with open` 语句读取了 `regions.txt` 和 `incomes.txt` 两个文件,并将它们分别存储在 `regions` 和 `incomes` 两个列表里。其中,`regions` 列表存储了各个地区的名称,`incomes` 列表存储了各个地区的收入数据。
然后,我们使用 matplotlib 库的 `plt.bar` 函数绘制了直方图。其中,`plt.bar` 函数的第一个参数是一个整数列表,表示每个柱状图的位置;第二个参数是一个浮点数列表,表示每个柱状图的高度;第三个参数是一个字符串列表,表示每个柱状图的标签。我们将 `regions` 列表作为标签,并将 `incomes` 列表中的数据作为高度,这样就可以绘制出各个地区的收入情况了。
最后,我们使用 matplotlib 库的其他函数设置了图表的标题、坐标轴标签等参数,并使用 `plt.show` 函数展示了图表。
相关推荐
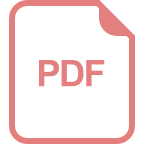
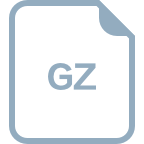
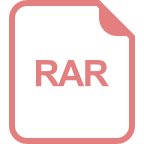












