用Java编写一个Student类 属性: (1学号; (2) 姓名 (3)成绩 方法: (1)打招呼:sayHello()方法:直接输出学号姓名,无返回值; (2) 会计算: compurtsum (方法:计算总成绩,各科成绩为方法的参数,方法有
时间: 2024-01-22 07:21:07 浏览: 184
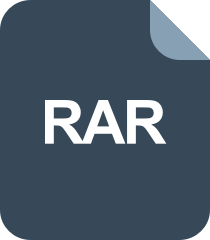
设计一个学生类Student(学生学号、姓名、数学、英语、计算机成绩;)

返回值)。
代码如下:
```java
public class Student {
private String studentId;
private String name;
private int score;
public Student(String studentId, String name, int score) {
this.studentId = studentId;
this.name = name;
this.score = score;
}
public void sayHello() {
System.out.println("Hello, my name is " + name + ", and my student ID is " + studentId);
}
public int computeSum(int mathScore, int englishScore, int chineseScore) {
return mathScore + englishScore + chineseScore;
}
}
```
在这个类中,我们定义了三个属性:学号、姓名和成绩。我们还定义了两个方法:sayHello(),它将输出学生的姓名和学号,而computeSum()将计算三个科目的总成绩,并将其作为返回值返回。我们使用构造函数来初始化学生的属性。
阅读全文
相关推荐
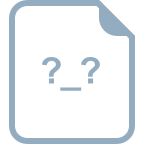
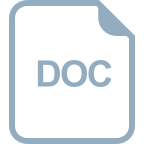











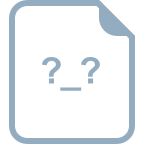




