class Node: def __init__(self, data=None, next=None): self.data = data self.next = nextclass LinkedList: def __init__(self): self.head = None def is_empty(self): return self.head is None def add(self, data): new_node = Node(data) new_node.next = self.head self.head = new_node def length(self): count = 0 current = self.head while current is not None: count += 1 current = current.next return count def insert(self, data, position): if position == 0: self.add(data) else: new_node = Node(data) current = self.head for i in range(position - 1): current = current.next new_node.next = current.next current.next = new_node def remove(self, position): if position == 0: self.head = self.head.next else: current = self.head for i in range(position - 1): current = current.next current.next = current.next.next def traverse(self): current = self.head while current is not None: print(current.data) current = current.next
时间: 2023-05-11 20:01:50 浏览: 217
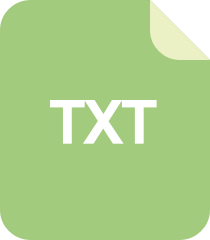
LinkedList的用法

这是一个链表的实现,包括节点类 Node 和链表类 LinkedList。其中,节点类 Node 包含数据和指向下一个节点的指针,链表类 LinkedList 包含头节点和一些基本操作,如判断链表是否为空、添加节点、获取链表长度、在指定位置插入节点、删除指定位置的节点和遍历链表。
阅读全文
相关推荐
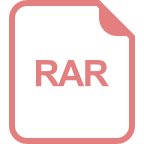
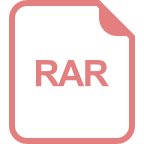













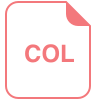
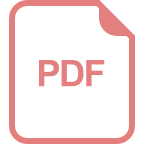