用C语言实现编写计算器程序,输入原表达式,输出表达式的值。要求能够支持括号,多位整数和小数的计算。
时间: 2024-05-08 14:17:39 浏览: 139
这是一个比较复杂的程序,需要用到数据结构和算法,下面是一个基本的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_STACK_SIZE 100
#define MAX_EXPR_SIZE 100
typedef enum {
LEFT_PAREN, RIGHT_PAREN, PLUS, MINUS, TIMES, DIVIDE, NUMBER, END
} Token;
int stack[MAX_STACK_SIZE];
int top = -1;
Token getToken(char *expr, int *pos);
void push(int x);
int pop();
int isFull();
int isEmpty();
int evaluate(char *expr);
int main() {
char expr[MAX_EXPR_SIZE];
printf("请输入表达式:");
scanf("%s", expr);
int result = evaluate(expr);
printf("结果为:%d\n", result);
return 0;
}
Token getToken(char *expr, int *pos) {
char c = expr[(*pos)++];
while (isspace(c)) {
c = expr[(*pos)++];
}
switch (c) {
case '(':
return LEFT_PAREN;
case ')':
return RIGHT_PAREN;
case '+':
return PLUS;
case '-':
return MINUS;
case '*':
return TIMES;
case '/':
return DIVIDE;
case '\0':
return END;
default:
if (isdigit(c)) {
int num = c - '0';
while (isdigit(expr[*pos])) {
num = num * 10 + (expr[(*pos)++] - '0');
}
if (expr[*pos] == '.') {
(*pos)++;
double decimal = 0.1;
while (isdigit(expr[*pos])) {
num = num + (expr[(*pos)++] - '0') * decimal;
decimal *= 0.1;
}
}
return NUMBER;
} else {
printf("Error: Invalid character\n");
exit(1);
}
}
}
void push(int x) {
if (isFull()) {
printf("Error: Stack overflow\n");
exit(1);
}
stack[++top] = x;
}
int pop() {
if (isEmpty()) {
printf("Error: Stack underflow\n");
exit(1);
}
return stack[top--];
}
int isFull() {
return top == MAX_STACK_SIZE - 1;
}
int isEmpty() {
return top == -1;
}
int evaluate(char *expr) {
int pos = 0;
Token token = getToken(expr, &pos);
while (token != END) {
if (token == NUMBER) {
push(atof(expr + pos - 1));
} else if (token == PLUS || token == MINUS || token == TIMES || token == DIVIDE) {
int right = pop();
int left = pop();
int result;
switch (token) {
case PLUS:
result = left + right;
break;
case MINUS:
result = left - right;
break;
case TIMES:
result = left * right;
break;
case DIVIDE:
result = left / right;
break;
}
push(result);
} else if (token == LEFT_PAREN) {
push(token);
} else if (token == RIGHT_PAREN) {
while (stack[top] != LEFT_PAREN) {
int right = pop();
int left = pop();
int result;
switch (pop()) {
case PLUS:
result = left + right;
break;
case MINUS:
result = left - right;
break;
case TIMES:
result = left * right;
break;
case DIVIDE:
result = left / right;
break;
}
push(result);
}
pop(); // 弹出左括号
}
token = getToken(expr, &pos);
}
return pop();
}
```
该程序使用了一个栈来保存运算符和操作数,可以处理加、减、乘、除、括号以及多位整数和小数。在计算表达式的过程中,会不断从字符串中读取出下一个 Token,然后根据 Token 类型进行相应的计算操作。
阅读全文
相关推荐
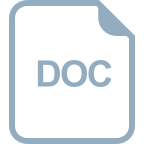
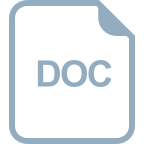
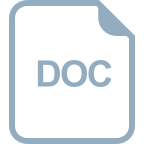
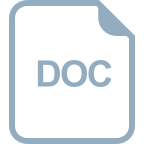








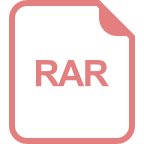
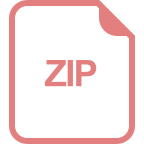
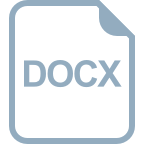
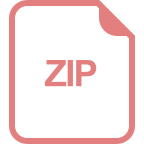
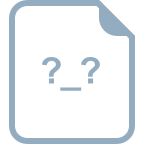
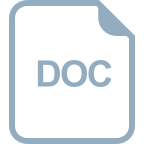
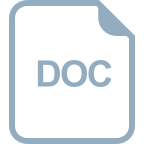