python将数值换成字符串
时间: 2023-08-08 20:08:51 浏览: 44
要将数值转换为字符串,可以使用内置的 `str()` 函数。例如:
```python
num = 123
num_str = str(num)
print(num_str)
```
输出:
```
'123'
```
如果你想将数值转换为不同进制的字符串,可以使用 `bin()`、`oct()` 和 `hex()` 函数。例如:
```python
num = 42
num_bin = bin(num)
num_oct = oct(num)
num_hex = hex(num)
print(num_bin, num_oct, num_hex)
```
输出:
```
'0b101010' '0o52' '0x2a'
```
相关问题
python将字符串换成数值类型
在 Python 中,有三种常见的将字符串转换为数值类型的方法:
1. int() 函数:将字符串转换为整数类型。
例如:
```python
num_str = '123'
num_int = int(num_str)
print(type(num_int)) # <class 'int'>
```
2. float() 函数:将字符串转换为浮点数类型。
例如:
```python
num_str = '3.14'
num_float = float(num_str)
print(type(num_float)) # <class 'float'>
```
3. eval() 函数:将字符串作为表达式求值,并返回结果。
例如:
```python
num_str = '3.14'
num_eval = eval(num_str)
print(type(num_eval)) # <class 'float'>
```
注意:eval() 函数会执行字符串中的任何表达式,因此在使用时要格外小心,确保字符串中不包含恶意代码。
python使用numpy库,将矩阵中每个数值转换成字符串
你可以使用numpy库的astype()方法将矩阵中每个数值转换成字符串。具体代码如下:
import numpy as np
# 定义一个矩阵
mat = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# 将矩阵中每个数值转换成字符串
str_mat = mat.astype(str)
# 打印转换后的字符串矩阵
print(str_mat)
相关推荐
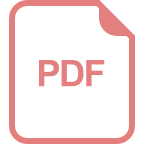
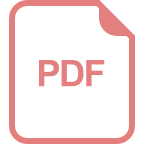
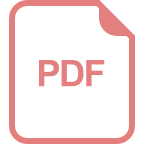












