const getInfo=()=>{ axios.get("http://localhost:3000/posts").then((res)=>{ console.log(res.data) }).catch((error)=>{ console.log(error) }) }报跨域错误
时间: 2024-03-11 19:46:53 浏览: 14
这是因为在使用axios进行请求时,请求的地址与当前页面的地址不在同一个域名下。浏览器出于安全考虑,禁止了这种跨域请求。你可以在服务端设置响应头,允许跨域请求。
可以在服务端设置响应头来允许跨域请求,例如:
```
app.use((req, res, next) => {
res.header('Access-Control-Allow-Origin', '*');
res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization');
res.header('Access-Control-Allow-Methods', 'POST, GET, OPTIONS');
next();
});
```
其中,`Access-Control-Allow-Origin`表示允许跨域请求的域名,`*`表示允许所有域名请求。`Access-Control-Allow-Headers`表示允许请求的头部信息,`Content-Type`和`Authorization`是常用的头部信息。`Access-Control-Allow-Methods`表示允许的请求方法,`POST`, `GET`, `OPTIONS`是常用的请求方法。
相关问题
修改代码 const add=()=>{ axios.post("/shelf/add",{ user_id:localStorage.getItem("id"), book_id:route.query.bookId }).then((res)=>{ const data = res.data console.log(data) }) }
这段代码是一个使用 axios 发送 POST 请求的函数,请求地址是 `/shelf/add`,请求参数是当前用户的 id 和书本的 id。当请求成功后,会将响应数据打印到控制台。如果要修改这个函数,可以根据实际需求修改请求地址和请求参数,如下所示:
```javascript
const add = () => {
axios.post("/api/add-to-cart", {
userId: localStorage.getItem("userId"),
productId: route.query.productId,
quantity: 1
}).then((res) => {
const data = res.data;
console.log(data);
});
};
```
这个例子中,我们将请求地址改为了 `/api/add-to-cart`,请求参数包括了用户的 id、产品的 id 和购买数量,用于向购物车添加商品。你可以根据具体的业务需求修改这些参数。
const getInfo=()=>{ axios.get("http://localhost:3000/posts",{ withCredentials: true }).then((res)=>{ console.log(res.data) }).catch((error)=>{ console.log(error) }) }服务器端设置了 res.header('Access-Control-Allow-Origin', '*'); res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization'); res.header('Access-Control-Allow-Methods', 'POST, GET, OPTIONS');还是报跨域错误
如果你已经在服务端设置了跨域响应头并且在axios请求时添加了`withCredentials: true`参数,但仍然报跨域错误,可能是因为axios请求的地址或者端口号不正确,可以尝试检查一下。
还有一种可能性是,如果你的服务端是使用Node.js搭建的,并且使用了`http`模块来创建服务器,那么你需要使用`cors`中间件来解决跨域问题。可以使用以下命令来安装`cors`:
```
npm install cors --save
```
然后在服务端代码中使用`cors`中间件,例如:
```
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors());
// 其他路由和中间件
app.listen(3000, () => {
console.log('server is running at http://localhost:3000');
});
```
这样就可以解决跨域问题了。
相关推荐
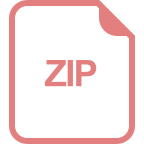
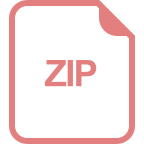
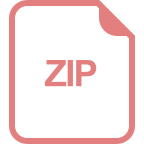













