c++ 根据qrcode字符串创建一个子窗口把qrcode生成图片显示在窗口上 不使用qt
时间: 2023-12-31 11:04:36 浏览: 132
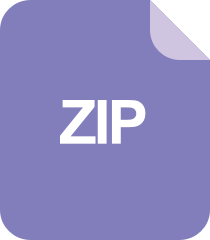
QT利用C++生成二维码qrcode

你可以使用第三方库 `qrcodegen` 来生成 QR Code 图片,然后再使用 Windows API 中的 GDI+ 库来将图片显示在窗口上。以下是一个示例程序,可以参考一下:
1. 下载 `qrcodegen.hpp` 文件,可以从 [GitHub](https://github.com/nayuki/QR-Code-generator) 上获取。
2. 将 `qrcodegen.hpp` 文件添加到你的项目中。
3. 使用 `qrcodegen` 库生成 QR Code 图片,并将图片显示在窗口上。
```c++
#include <windows.h>
#include <gdiplus.h>
#include "qrcodegen.hpp"
#pragma comment(lib, "Gdiplus.lib")
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
// 初始化 GDI+
Gdiplus::GdiplusStartupInput gdiplusStartupInput;
ULONG_PTR gdiplusToken;
Gdiplus::GdiplusStartup(&gdiplusToken, &gdiplusStartupInput, NULL);
// 创建窗口
HWND hwnd;
WNDCLASS wc = { 0 };
wc.lpfnWndProc = WndProc;
wc.hInstance = hInstance;
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszClassName = L"QRCodeWindow";
if (!RegisterClass(&wc))
return 1;
hwnd = CreateWindow(wc.lpszClassName, L"QR Code", WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, 640, 480, NULL, NULL, hInstance, NULL);
if (!hwnd)
return 2;
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
// 生成 QR Code 图片
qrcodegen::QrCode qr = qrcodegen::QrCode::encodeText("Hello, world!", qrcodegen::QrCode::Ecc::LOW);
const int size = qr.getSize();
const int border = 4;
const int imageSize = size * 10 + border * 2;
Gdiplus::Bitmap bitmap(imageSize, imageSize, PixelFormat32bppRGB);
Gdiplus::Graphics graphics(&bitmap);
graphics.Clear(Gdiplus::Color(255, 255, 255, 255));
for (int y = 0; y < size; y++) {
for (int x = 0; x < size; x++) {
if (qr.getModule(x, y)) {
const int left = x * 10 + border;
const int top = y * 10 + border;
graphics.FillRectangle(&Gdiplus::SolidBrush(Gdiplus::Color(0, 0, 0)), left, top, 10, 10);
}
}
}
// 进入消息循环
MSG msg;
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
// 释放 GDI+
Gdiplus::GdiplusShutdown(gdiplusToken);
return (int)msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
switch (message)
{
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
// 使用 GDI+ 绘制图片
Gdiplus::Graphics graphics(hdc);
Gdiplus::Bitmap bitmap(L"qrcode.png", FALSE);
graphics.DrawImage(&bitmap, 0, 0);
EndPaint(hwnd, &ps);
break;
}
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, message, wParam, lParam);
}
return 0;
}
```
这个程序会创建一个窗口,然后在窗口上显示生成的 QR Code 图片。你可以根据需要修改窗口的大小和位置,以及 QR Code 图片的内容和纠错级别。
阅读全文
相关推荐
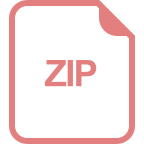
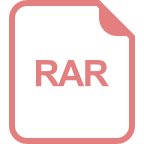















