python查找两个数组中相同的元素
时间: 2023-08-04 07:35:40 浏览: 146
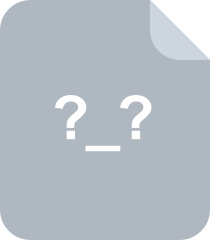
搜索一维数组中相同的值
可以使用Python内置函数set()和&运算符来查找两个数组中相同的元素。
下面是一个示例代码:
```python
arr1 = [1, 2, 3, 4, 5]
arr2 = [4, 5, 6, 7, 8]
set1 = set(arr1)
set2 = set(arr2)
common_elements = set1 & set2
print(common_elements)
```
输出结果为:
```
{4, 5}
```
说明数组arr1和arr2中都包含元素4和5。
阅读全文
相关推荐

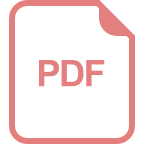
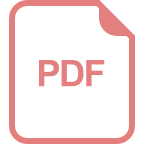
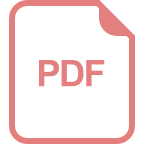
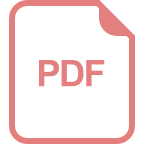
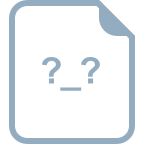









