WARNING: Mac address to reach destination not found. Using broadcast
时间: 2024-04-06 10:33:46 浏览: 14
这是一个网络通信中的警告信息,意思是无法找到用于到达目标地址的 MAC 地址,因此使用广播方式发送信息。在网络通信中,每个设备都有一个唯一的 MAC 地址,用于标识设备的物理地址。当一台设备需要发送数据到另一台设备时,需要知道目标设备的 MAC 地址才能够正确地发送数据。如果无法找到目标设备的 MAC 地址,就需要使用广播方式发送数据,这样所有与网络相连的设备都会接收到该数据包。
相关问题
消除WARNING: more Mac address to reach destination not found. Using broadcast
这个警告一般是在你的网络中存在多个接口或者子网时出现的。当你的计算机要发送一个数据包到一个不在同一个子网中的目标地址时,它需要使用ARP协议来查询目标地址的MAC地址。如果在你的网络中存在多个不同的子网或者接口,计算机就会尝试使用广播地址发送ARP请求,以确定目标地址的MAC地址。当计算机无法找到目标地址的MAC地址时,就会发出上述警告。
要消除这个警告,你可以尝试以下方法:
1. 确保你的计算机连接到正确的子网和接口上。
2. 确认你的网络配置是否正确,包括IP地址、子网掩码、网关等等。
3. 如果你的计算机连接到了多个网络或者接口上,可以尝试禁用其中的一些接口,或者使用路由表来控制数据包的传输。
4. 如果你使用的是虚拟机或者容器等隔离技术,需要设置正确的网络配置和路由表,以确保数据包能够正确地传输。
使用scapy发送icmp报文,出现WARNING: Mac address to reach destination not found. Using broadcast.错误,请问如何解决
这个警告是因为在发送 ICMP 报文时,Scapy 没有找到目标主机的 MAC 地址,所以使用广播方式发送。这通常是因为目标主机不在同一局域网内,或者目标主机的 ARP 缓存已经过期。
要解决这个问题,可以手动发送 ARP 请求来获取目标主机的 MAC 地址。可以使用以下代码片段:
```python
from scapy.all import *
# 目标主机的 IP 地址
ip = "192.168.1.1"
# 发送 ARP 请求
arp = ARP(pdst=ip)
ether = Ether(dst="ff:ff:ff:ff:ff:ff")
packet = ether/arp
result = srp(packet, timeout=3, verbose=False)[0]
# 获取目标主机的 MAC 地址
for sent, received in result:
return received.hwsrc
```
这段代码发送一个 ARP 请求,获取目标主机的 MAC 地址,并返回 MAC 地址。然后你可以使用这个 MAC 地址发送 ICMP 报文。
相关推荐
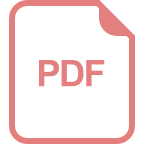













