tensorflow将图片导入
时间: 2023-07-09 17:19:01 浏览: 214
你可以使用TensorFlow中的tf.keras.preprocessing.image模块来导入图片。以下是一些基本步骤:
1. 导入模块
``` python
import tensorflow as tf
from tensorflow.keras.preprocessing.image import load_img, img_to_array
```
2. 加载图片
``` python
img = load_img('path/to/image.jpg')
```
其中,'path/to/image.jpg'是图片的路径。
3. 将图片转换为TensorFlow张量
``` python
img_array = img_to_array(img)
```
现在,你已经成功地将图片导入到TensorFlow中了。你可以将其用作输入数据,以训练或测试模型。
相关问题
tensorflow加载图片
在 TensorFlow 中加载图片可以使用 `tf.keras.preprocessing.image.load_img` 函数。具体步骤如下:
1. 导入相关库:
```python
import tensorflow as tf
from tensorflow.keras.preprocessing.image import load_img
```
2. 加载图片:
```python
img = load_img('path/to/image.jpg', target_size=(224, 224))
```
其中,`path/to/image.jpg` 是图片的路径,`target_size=(224, 224)` 是将图片缩放为指定的大小。`load_img` 函数将返回一个 PIL 图像对象。
3. 将 PIL 图像对象转换为 NumPy 数组:
```python
img_array = tf.keras.preprocessing.image.img_to_array(img)
```
这样就可以将 PIL 图像对象转换为 NumPy 数组,便于后续处理。
完整代码如下:
```python
import tensorflow as tf
from tensorflow.keras.preprocessing.image import load_img
# 加载图片
img = load_img('path/to/image.jpg', target_size=(224, 224))
# 将 PIL 图像对象转换为 NumPy 数组
img_array = tf.keras.preprocessing.image.img_to_array(img)
# 打印图像数组的形状
print(img_array.shape)
```
注意,在加载图片之前需要确保已经安装了 PIL 或 Pillow 库。如果没有安装,可以通过以下命令安装:
```bash
pip install pillow
```
tensorflow读取图片训练
使用tensorflow读取图片进行训练可以通过以下步骤完成。首先,需要导入tensorflow库,并且使用tensorflow中的函数将图片读取进来。然后,可以对图片进行预处理,例如调整大小、裁剪、归一化等操作。接下来,需要将处理后的图片数据与对应的标签组合成训练集,这样可以训练出一个模型,能够准确地识别不同的图片。训练的过程中,可以利用tensorflow提供的各种优化器和损失函数来帮助模型不断优化,从而提高识别的准确性。训练完成后,可以使用测试集来验证模型的准确性,如果表现不佳,还可以通过调整训练集的大小、增加训练次数或者修改模型的架构等方法来进一步优化模型。通过以上步骤,就可以使用tensorflow读取图片进行训练,从而实现对图片的准确识别,适用于各种图像识别的应用场景。
阅读全文
相关推荐
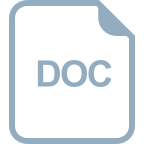
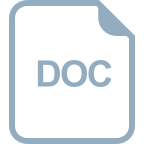
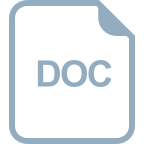
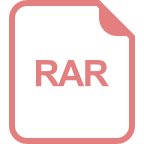


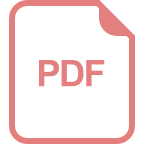
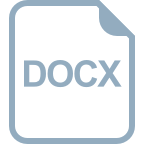
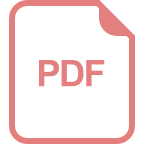
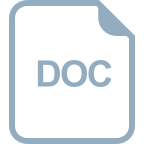
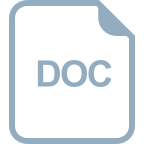
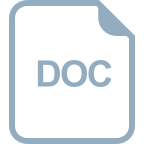
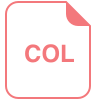


