协议分析器分析以太网C++编写代码
时间: 2023-08-12 19:24:49 浏览: 43
以下是一个简单的C++代码示例,用于解析以太网协议中的数据帧:
```c++
#include <iostream>
#include <cstring>
#include <netinet/in.h>
#include <netinet/if_ether.h>
using namespace std;
int main()
{
// 假设我们已经从网络中获取了一个数据帧,保存在buffer中
char buffer[1024] = {0};
// 解析以太网头
struct ether_header *eth_header = (struct ether_header*)buffer;
// 打印源MAC地址和目标MAC地址
cout << "Source MAC: " << ether_ntoa((struct ether_addr*)eth_header->ether_shost) << endl;
cout << "Destination MAC: " << ether_ntoa((struct ether_addr*)eth_header->ether_dhost) << endl;
// 根据以太网类型,判断上层协议
unsigned short ether_type = ntohs(eth_header->ether_type);
if (ether_type == ETHERTYPE_IP) {
// 解析IP数据报
cout << "IP Protocol" << endl;
} else if (ether_type == ETHERTYPE_ARP) {
// 解析ARP数据帧
cout << "ARP Protocol" << endl;
} else if (ether_type == ETHERTYPE_VLAN) {
// 解析VLAN数据帧
cout << "VLAN Protocol" << endl;
} else {
// 其他协议
cout << "Unknown Protocol" << endl;
}
return 0;
}
```
需要注意的是,实际的以太网协议分析器需要考虑更多的情况,例如VLAN标签、帧的长度等。同时,也需要考虑到协议的各种变化和特殊情况,以确保分析器能够正确地处理各种数据帧。
相关推荐
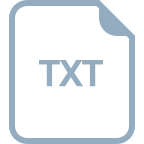
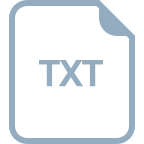
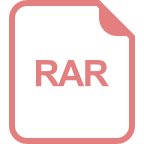














