C# winform 不同版本的excel表导入datagridview
时间: 2023-07-30 08:05:51 浏览: 118
C# WinForm 可以使用 Microsoft.Office.Interop.Excel 库来实现 Excel 表导入到 DataGridView 中。不同版本的 Excel 文件导入方式略有不同,下面是两种常见的版本的导入方式:
1. Excel 2007 及以上版本
```csharp
using System.Data;
using System.Data.OleDb;
using System.Windows.Forms;
private void btnImport_Click(object sender, EventArgs e)
{
OpenFileDialog openFileDialog = new OpenFileDialog();
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
string filePath = openFileDialog.FileName;
string extension = Path.GetExtension(filePath);
string header = "YES";
string conStr = string.Empty;
DataTable dt = new DataTable();
switch (extension)
{
case ".xls": // Excel 97-03
conStr = string.Format("Provider=Microsoft.Jet.OLEDB.4.0;Data Source={0};Extended Properties=\"Excel 8.0;HDR={1}\"", filePath, header);
break;
case ".xlsx": // Excel 07 or higher
conStr = string.Format("Provider=Microsoft.ACE.OLEDB.12.0;Data Source={0};Extended Properties=\"Excel 12.0;HDR={1}\"", filePath, header);
break;
}
using (OleDbConnection con = new OleDbConnection(conStr))
{
con.Open();
DataTable schemaTable = con.GetOleDbSchemaTable(OleDbSchemaGuid.Tables, new object[] { null, null, null, "TABLE" });
string sheetName = schemaTable.Rows[0]["TABLE_NAME"].ToString();
string query = string.Format("SELECT * FROM [{0}]", sheetName);
using (OleDbDataAdapter adapter = new OleDbDataAdapter(query, con))
{
adapter.Fill(dt);
}
}
dataGridView1.DataSource = dt;
}
}
```
2. Excel 97-03 版本
```csharp
using System.Data;
using System.Data.OleDb;
using System.Windows.Forms;
private void btnImport_Click(object sender, EventArgs e)
{
OpenFileDialog openFileDialog = new OpenFileDialog();
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
string filePath = openFileDialog.FileName;
string header = "YES";
string conStr = string.Format("Provider=Microsoft.Jet.OLEDB.4.0;Data Source={0};Extended Properties=\"Excel 8.0;HDR={1}\"", filePath, header);
DataTable dt = new DataTable();
using (OleDbConnection con = new OleDbConnection(conStr))
{
con.Open();
DataTable schemaTable = con.GetOleDbSchemaTable(OleDbSchemaGuid.Tables, new object[] { null, null, null, "TABLE" });
string sheetName = schemaTable.Rows[0]["TABLE_NAME"].ToString();
string query = string.Format("SELECT * FROM [{0}]", sheetName);
using (OleDbDataAdapter adapter = new OleDbDataAdapter(query, con))
{
adapter.Fill(dt);
}
}
dataGridView1.DataSource = dt;
}
}
```
阅读全文
相关推荐
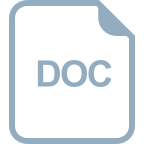
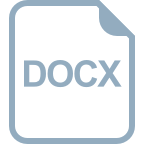















