uniapp,获取微信小程序用户信息
时间: 2023-10-24 16:05:18 浏览: 169
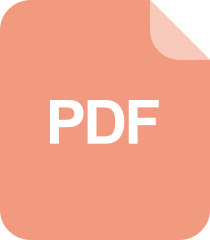
微信小程序 - 获取用户信息

要在uniapp中获取微信小程序用户信息,需要进行以下步骤:
1. 在微信公众平台中,开启“用户信息”权限。
2. 使用uniapp的wx.getUserInfo()方法获取用户信息。
3. 在打开小程序时,需要请求用户授权,获取用户信息。
下面是示例代码:
```
// 打开小程序时,请求用户授权
wx.getUserProfile({
desc: '用于完善会员资料',
success: res => {
console.log(res)
// 获取用户信息成功
// 可以将用户信息存储到全局变量中,方便后续使用
},
fail: res => {
console.log(res)
// 获取用户信息失败
}
});
```
需要注意的是,getUserProfile方法需要在微信客户端版本6.5.9及以上才能使用。如果用户的微信版本过低,需要引导用户升级微信客户端。
阅读全文
相关推荐
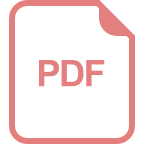















