@ApiOperation(value = "根据名称和年龄段查找用户") // 修改API文档描述 @ApiImplicitParams(value = { @ApiImplicitParam(name = "名称", value = "年龄段", dataTypeClass = String.class) }) @GetMapping ("/find") public UserInfo findDeviceInfo(@RequestParam String name,@RequestParam int age) throws Exception { return deviceService.findUserInfoByIdAndName(age, name); }通过控制层代码编写出业务层代码
时间: 2024-02-25 14:57:04 浏览: 63
根据你提供的控制层代码,我们可以编写出相应的业务层代码,如下所示:
```java
@Service
public class DeviceService {
@Autowired
private UserRepository userRepository;
public UserInfo findUserInfoByIdAndName(int age, String name) {
return userRepository.findByNameAndAge(name, age);
}
}
```
其中,`UserRepository` 是一个 DAO 层的接口,用于从数据库中获取用户信息。可以使用 Spring Data JPA 等 ORM 框架来实现该接口。在该接口中,应该定义一个 `findByNameAndAge` 方法,用于根据用户的姓名和年龄段查找用户信息。具体实现方式可以参考 ORM 框架的文档。
在业务层代码中,我们注入了 `UserRepository` 对象,并且定义了一个 `findUserInfoByIdAndName` 方法,用于调用 `UserRepository` 中的 `findByNameAndAge` 方法来获取用户信息。注意,参数列表的顺序与控制层代码中的相反,这是因为参数顺序与 API 文档描述中的顺序一致。
这样,我们就可以根据控制层代码编写出相应的业务层代码了。
相关问题
@ApiOperation(value = "根据名称和年龄段查找用户") // 修改API文档描述 @ApiImplicitParams(value = { @ApiImplicitParam(name = "名称", value = "年龄段", dataTypeClass = String.class) }) @GetMapping ("/find") public UserInfo findDeviceInfo(@RequestParam String name,@RequestParam int age) throws Exception { return deviceService.findUserInfoByIdAndName(age, name); }通过控制层代码编写出业务层代码
以下是通过控制层代码编写出的业务层代码:
```java
@Service
public class DeviceService {
@Autowired
private UserInfoMapper userInfoMapper;
public UserInfo findUserInfoByIdAndName(int age, String name) throws Exception {
if (StringUtils.isBlank(name)) {
throw new Exception("名称不能为空");
}
if (age < 0 || age > 120) {
throw new Exception("年龄超出范围");
}
UserInfoExample example = new UserInfoExample();
UserInfoExample.Criteria criteria = example.createCriteria();
criteria.andNameEqualTo(name);
criteria.andAgeBetween(age - 10, age + 10);
List<UserInfo> list = userInfoMapper.selectByExample(example);
if (list == null || list.size() == 0) {
throw new Exception("未找到符合条件的用户");
}
return list.get(0);
}
}
```
这段代码是业务层的代码,它通过调用userInfoMapper中的selectByExample方法来查找符合条件的用户信息。在方法中添加了参数校验,如果名称为空、年龄超出范围或未找到符合条件的用户则抛出异常。
解释以下代码:@TycloudOperation(ApiLevel = UserType.AGENCY,needAuth = false) @ApiOperation(httpMethod = "Post",value = "导入", notes = "导入") @PostMapping(value = "/import") public void importTplImportModel(@RequestPart("file") MultipartFile file) throws Exception { List<TplImportModel> tplImportModels = ExcelUtils.readMultipartFile(file,TplImportModel.class); for(TplImportModel tplImportModel : tplImportModels){ System.out.println(tplImportModel.toString()); } },调用该接口时,提示数据类型转换错误,怎么改
这段代码是一个用于文件导入的接口,使用了注解@TycloudOperation和@ApiOperation来指定接口的操作等级和描述信息,使用了@PostMapping来指定接口的请求方法为POST,使用@RequestPart来接收文件上传。代码中使用了ExcelUtils工具类来读取Excel文件,并将数据转换为TplImportModel类的List集合。
如果调用该接口时提示数据类型转换错误,可以考虑检查TplImportModel类中的属性与Excel文件中的列名是否一致。如果列名与属性名不一致,可以在TplImportModel类中使用@ExcelProperty注解来指定列名与属性的映射关系,例如:
```
public class TplImportModel {
@ExcelProperty(value = "名称", index = 0)
private String name;
@ExcelProperty(value = "年龄", index = 1)
private Integer age;
// ...
}
```
其中@ExcelProperty注解中的value属性指定Excel文件中的列名,index属性指定列的下标。这样就可以正确地将Excel文件中的数据转换为TplImportModel对象了。
阅读全文
相关推荐
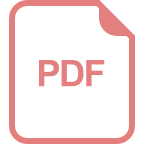
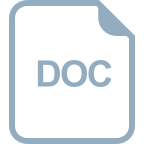
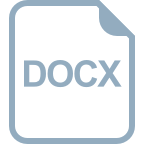












