QT5.9.12 如何把一个中文,英文混合字符串输出到一个二进制文件里,要用Unicode ,英文为1byte, 中文为2byte,文件大小固定为1024byte,不够字节数补0,要求要用QTextStream方法,
时间: 2024-03-10 19:46:04 浏览: 57
首先,我们需要使用QFile类创建一个二进制文件,并使用QTextStream类将数据写入文件。具体实现步骤如下:
1. 创建QFile对象并打开文件
```c++
QFile file("test.bin");
if (!file.open(QIODevice::WriteOnly | QIODevice::Truncate)) {
qDebug() << "Failed to open file";
return;
}
```
2. 创建QTextStream对象并设置编码格式为Unicode
```c++
QTextStream out(&file);
out.setCodec("UTF-16");
```
3. 将字符串按照要求写入文件中
```c++
QString str = "Hello, 你好!";
int bytesLeft = 1024; // 文件大小固定为1024byte
int strLen = str.length();
for (int i = 0; i < strLen && bytesLeft > 0; i++) {
QChar ch = str[i];
ushort code = ch.unicode();
if (code <= 0x7F) {
// 英文字符,1byte
out << (quint8)code;
bytesLeft -= 1;
} else {
// 中文字符,2byte
if (bytesLeft >= 2) {
out << code;
bytesLeft -= 2;
} else {
// 不够字节数,补0
out << (quint16)0;
bytesLeft -= 2;
}
}
}
// 文件不够字节数,补0
while (bytesLeft > 0) {
out << (quint16)0;
bytesLeft -= 2;
}
```
4. 关闭文件
```c++
file.close();
```
完整代码如下:
```c++
#include <QCoreApplication>
#include <QFile>
#include <QTextStream>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QFile file("test.bin");
if (!file.open(QIODevice::WriteOnly | QIODevice::Truncate)) {
qDebug() << "Failed to open file";
return -1;
}
QTextStream out(&file);
out.setCodec("UTF-16");
QString str = "Hello, 你好!";
int bytesLeft = 1024;
int strLen = str.length();
for (int i = 0; i < strLen && bytesLeft > 0; i++) {
QChar ch = str[i];
ushort code = ch.unicode();
if (code <= 0x7F) {
// 英文字符,1byte
out << (quint8)code;
bytesLeft -= 1;
} else {
// 中文字符,2byte
if (bytesLeft >= 2) {
out << code;
bytesLeft -= 2;
} else {
// 不够字节数,补0
out << (quint16)0;
bytesLeft -= 2;
}
}
}
// 文件不够字节数,补0
while (bytesLeft > 0) {
out << (quint16)0;
bytesLeft -= 2;
}
file.close();
return a.exec();
}
```
阅读全文
相关推荐







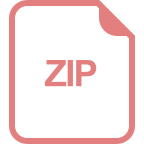




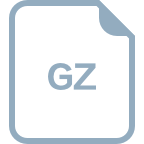
