matlab pso
时间: 2023-09-23 19:09:13 浏览: 73
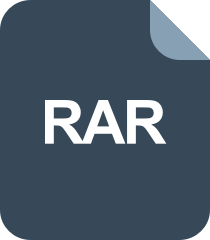
基于Matlab pso
PSO (Particle Swarm Optimization) is a popular optimization technique used in MATLAB. It is a stochastic optimization method that is inspired by the behavior of bird flocks or fish schools. In PSO, a swarm of particles moves through the search space and updates their positions based on their own best solution and the global best solution found by the swarm.
To implement PSO in MATLAB, you can follow these steps:
1. Define the objective function that you want to optimize.
2. Set the parameters of the algorithm, such as the number of particles, the maximum number of iterations, and the inertia weight.
3. Initialize the swarm particles with random positions and velocities.
4. Evaluate the objective function for each particle and determine their personal best position.
5. Determine the global best position of the swarm.
6. Update the velocity and position of each particle based on their personal and global best positions.
7. Check if the stopping criteria are met (e.g., maximum number of iterations reached or convergence achieved).
8. Repeat steps 4-7 until the stopping criteria are met.
Here is an example MATLAB code for PSO:
% Define the objective function
fun = @(x) x(1)^2 + x(2)^2;
% Set the parameters of the algorithm
num_particles = 50;
max_iterations = 100;
inertia_weight = 0.8;
c1 = 2;
c2 = 2;
% Initialize the swarm particles
positions = rand(num_particles, 2);
velocities = rand(num_particles, 2);
% Evaluate the objective function and determine personal best positions
personal_best = positions;
personal_best_fitness = arrayfun(fun, positions);
% Determine global best position
[global_best_fitness, global_best_index] = min(personal_best_fitness);
global_best = personal_best(global_best_index, :);
% Main loop
for i = 1:max_iterations
% Update velocity and position of particles
rand1 = rand(num_particles, 2);
rand2 = rand(num_particles, 2);
velocities = inertia_weight*velocities + c1*rand1.*(personal_best - positions) + c2*rand2.*(global_best - positions);
positions = positions + velocities;
% Evaluate objective function and update personal best position
fitness = arrayfun(fun, positions);
update_indices = fitness < personal_best_fitness;
personal_best(update_indices, :) = positions(update_indices, :);
personal_best_fitness(update_indices) = fitness(update_indices);
% Update global best position
[min_fitness, min_index] = min(personal_best_fitness);
if min_fitness < global_best_fitness
global_best_fitness = min_fitness;
global_best = personal_best(min_index, :);
end
% Check stopping criteria
if i == max_iterations
disp('Maximum iterations reached');
elseif global_best_fitness < 1e-6
disp('Convergence achieved');
break;
end
end
disp(['Global best position: (' num2str(global_best(1)) ', ' num2str(global_best(2)) ')']);
disp(['Global best fitness: ' num2str(global_best_fitness)]);
阅读全文
相关推荐
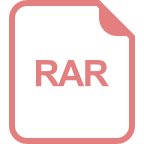
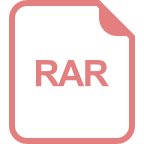
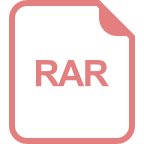
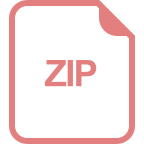
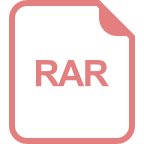
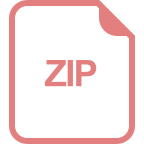
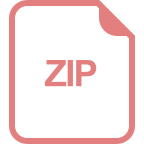
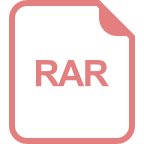
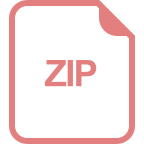
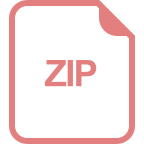
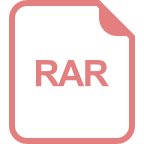
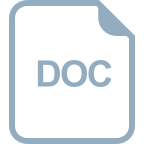
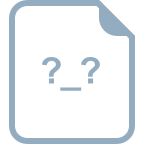
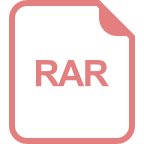