RuntimeWarning: invalid value encountered in sqrt colors[s] = cmap(np.sqrt((pop - vmin) / (vmax - vmin)))[:3]
时间: 2023-06-17 21:07:21 浏览: 61
这个警告是因为您在使用 np.sqrt() 函数时,传递了一个负数,导致返回了一个无效值(NaN)。您可以通过检查输入数据来避免这个问题,确保不会传递负数给 np.sqrt() 函数。另外,您可以使用 np.nan_to_num() 函数来将 NaN 值替换为 0 或其他值。例如,您可以将代码修改为:
```
import numpy as np
# some code here...
pop = np.array([3, -2, 4, 5, -1])
vmin, vmax = 0, 5
cmap = plt.get_cmap('Blues')
pop = np.nan_to_num(pop) # replace NaN with 0
colors = np.zeros((len(pop), 3))
for s, pop_val in enumerate(pop):
colors[s] = cmap(np.sqrt((pop_val - vmin) / (vmax - vmin)))[:3]
```
这样就可以避免这个警告了。
相关问题
RuntimeWarning: invalid value encountered in sqrt VI = np.sqrt(ratio)
这个警告是因为计算中出现了负数,导致计算平方根时出现了无效值(NaN)。可以通过检查计算中是否出现负数来解决这个问题。在这里,你可以在计算之前先检查 ratio 中是否存在负数,例如:
```python
def calculate_VI(EI, SI, RI):
EI = np.where(EI == -999, np.nan, EI).astype(np.float64)
SI = np.where(SI == -999, np.nan, SI).astype(np.float64)
RI = np.where(RI == -999, np.nan, RI).astype(np.float64)
numerator = EI * SI
denominator = 1 + RI
ratio = numerator / denominator
# 检查比值是否存在负值
if np.any(ratio < 0):
raise ValueError('Ratio contains negative value')
VI = np.sqrt(ratio)
return VI
```
在这里,如果 ratio 中存在负数,就抛出一个 ValueError 异常,提示计算结果不正确。这样可以及时发现问题并修复。
RuntimeWarning: invalid value encountered in sqrt rms = np.sqrt(np.mean(np.square(data)))
这个警告是因为计算RMS时,数据中可能会出现负值,导致取平方根时出现了负数,从而产生了无效值(NaN)。为了避免这个问题,可以将数据中的负数取绝对值后再计算RMS。
以下是修改后的示例代码:
```python
import pyaudio
import numpy as np
# 定义一些参数
RATE = 16000
CHUNK = 1024
VAD_THRESHOLD = 500
p = pyaudio.PyAudio()
stream = p.open(format=pyaudio.paInt16, channels=1, rate=RATE, input=True, frames_per_buffer=CHUNK)
print("* 开始录音...")
while True:
# 读取一段音频数据
data = stream.read(CHUNK)
data = np.frombuffer(data, dtype=np.int16)
# 计算音量
rms = np.sqrt(np.mean(np.square(np.abs(data))))
# 如果音量大于阈值,则认为有人在说话
if rms > VAD_THRESHOLD:
print("有人在说话!")
print("* 结束录音...")
stream.stop_stream()
stream.close()
p.terminate()
```
该代码使用`np.abs`函数将数据中的负数取绝对值,然后计算RMS。如果音量大于预设的阈值,就会输出“有人在说话!”的提示信息。
相关推荐
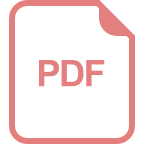
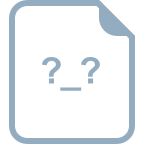
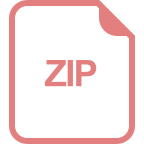












