1、使用LCD1602作为显示器。2、使用单片机定时器作为时间基准 3、使用独立按键,切换秒表和计时器功能 4、秒表模式下以0.01秒为单位显示变化,一个按键用于开始和停止。5、计时模式进行倒计时,使用组合按键调整计时时间长度,一秒为单位。计时模式下使用一个按键开始和停止计时,即使时间到蜂鸣器叫,使用该按键关闭提醒,重新回到设定的时间长度显示状态。怎么编写程序
时间: 2024-02-16 17:02:38 浏览: 25
以下是基于Arduino平台的程序示例,使用了LCD1602液晶显示屏和常见的4x4矩阵按键模块,你可以根据自己的硬件配置进行适当的修改:
```
#include <LiquidCrystal.h>
#define ROWS 4
#define COLS 4
LiquidCrystal lcd(12, 11, 5, 4, 3, 2); // 初始化LCD
const int buzzer = 6; // 蜂鸣器引脚
const int start_stop_button = 7; // 开始/停止按键引脚
const int mode_button = 8; // 模式切换按键引脚
const int adjust_button = 9; // 时间调整按键引脚
int mode = 0; // 记录当前模式(0为计时模式,1为秒表模式)
int state = 0; // 记录开始/停止状态(0为停止,1为运行)
unsigned long start_time = 0; // 记录计时开始时间
unsigned long elapsed_time = 0; // 记录经过的时间
unsigned long set_time = 0; // 记录设定的倒计时时间
unsigned long last_adjust_time = 0; // 上次时间调整的时间
int adjust_mode = 0; // 记录当前时间调整的模式(0为小时,1为分钟,2为秒)
void setup() {
lcd.begin(16, 2); // 初始化LCD
pinMode(buzzer, OUTPUT); // 设置蜂鸣器引脚为输出
pinMode(start_stop_button, INPUT_PULLUP); // 设置开始/停止按键为上拉输入
pinMode(mode_button, INPUT_PULLUP); // 设置模式切换按键为上拉输入
pinMode(adjust_button, INPUT_PULLUP); // 设置时间调整按键为上拉输入
attachInterrupt(digitalPinToInterrupt(start_stop_button), start_stop, FALLING); // 绑定开始/停止按键中断
attachInterrupt(digitalPinToInterrupt(mode_button), switch_mode, FALLING); // 绑定模式切换按键中断
attachInterrupt(digitalPinToInterrupt(adjust_button), adjust_time, FALLING); // 绑定时间调整按键中断
}
void loop() {
lcd.clear();
if (mode == 0) { // 计时模式
lcd.print("Count Down");
lcd.setCursor(0, 1);
if (state == 0) { // 停止状态
lcd.print("Set Time:");
lcd.print(format_time(set_time));
} else { // 运行状态
elapsed_time = millis() - start_time;
if (elapsed_time >= set_time * 1000) { // 时间到
state = 0;
tone(buzzer, 1000, 500); // 发出提示音
}
lcd.print("Remaining:");
lcd.print(format_time((set_time * 1000 - elapsed_time) / 1000));
}
} else { // 秒表模式
lcd.print("Stop Watch");
lcd.setCursor(0, 1);
if (state == 0) { // 停止状态
lcd.print("00:00:00.00");
} else { // 运行状态
lcd.print(format_time(elapsed_time / 10));
}
}
delay(100); // 刷新速度
}
void start_stop() {
if (mode == 0) { // 计时模式
if (state == 0) { // 停止状态
state = 1; // 进入运行状态
start_time = millis(); // 记录开始时间
} else { // 运行状态
state = 0; // 进入停止状态
}
} else { // 秒表模式
if (state == 0) { // 停止状态
state = 1; // 进入运行状态
start_time = millis() - elapsed_time; // 恢复上次的计时时间
} else { // 运行状态
state = 0; // 进入停止状态
elapsed_time = millis() - start_time; // 记录经过的时间
}
}
}
void switch_mode() {
mode = (mode + 1) % 2; // 切换模式
state = 0; // 进入停止状态
elapsed_time = 0; // 清零计时器
set_time = 0; // 清零倒计时时间
}
void adjust_time() {
if (millis() - last_adjust_time > 500) { // 防止按键抖动
if (mode == 0) { // 计时模式
if (state == 0) { // 停止状态
adjust_mode = (adjust_mode + 1) % 3; // 切换调整模式
} else { // 运行状态
tone(buzzer, 1000, 100); // 发出错误提示音
}
} else { // 秒表模式
if (state == 0) { // 停止状态
elapsed_time = 0; // 清零计时器
} else { // 运行状态
tone(buzzer, 1000, 100); // 发出错误提示音
}
}
last_adjust_time = millis(); // 记录上次调整时间
}
}
void adjust_value(int delta) {
switch (adjust_mode) {
case 0: // 调整小时
set_time += delta * 3600;
break;
case 1: // 调整分钟
set_time += delta * 60;
break;
case 2: // 调整秒钟
set_time += delta;
break;
}
if (set_time < 0) { // 防止倒计时时间为负
set_time = 0;
}
}
void format_time(unsigned long time) {
unsigned long h = time / 3600;
unsigned long m = (time % 3600) / 60;
unsigned long s = time % 60;
unsigned long ms = (time % 1000) / 10;
lcd.print(h < 10 ? "0" : "");
lcd.print(h);
lcd.print(":");
lcd.print(m < 10 ? "0" : "");
lcd.print(m);
lcd.print(":");
lcd.print(s < 10 ? "0" : "");
lcd.print(s);
lcd.print(".");
lcd.print(ms < 10 ? "0" : "");
lcd.print(ms);
}
```
这是一个简单的程序示例,你可以根据自己的需求进行适当的修改和完善。
相关推荐
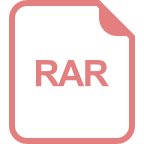
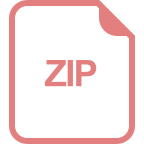














