aesutil.encrypt php
时间: 2023-08-23 16:05:01 浏览: 176
您想了解关于aesutil.encrypt在PHP中的用法吗?
aesutil.encrypt是一个加密工具类,用于在PHP中加密数据。具体的使用方法需要根据您所使用的aesutil.encrypt工具类的具体实现来确定。
一般情况下,您需要先引入aesutil.encrypt工具类,然后根据具体的加密算法和密钥,调用相应的加密方法进行加密。例如,使用AES算法进行加密,可以使用以下代码:
```
require_once('aesutil.php');
$aes = new AESUtil('your_secret_key');
$encrypted_data = $aes->encrypt('your_data');
```
其中,'your_secret_key'是您自定义的密钥,'your_data'是您要加密的数据。加密后的数据将存储在$encrypted_data变量中。
需要注意的是,加密算法和密钥的选择对于加密结果的安全性有着重要的影响。因此,在实际应用中,应该根据具体的安全需求选择合适的加密算法和密钥。
相关问题
springboot中的AESUtil.decrypt
在 Spring Boot 中,可以使用 AESUtil 类来进行 AES 加密和解密操作。具体实现步骤如下:
1. 导入依赖
在 pom.xml 文件中添加以下依赖:
```
<dependency>
<groupId>javax.crypto</groupId>
<artifactId>jce</artifactId>
<version>1.0.2</version>
</dependency>
```
2. 实现 AESUtil 类
在 utils 包中创建 AESUtil 类,在该类中实现 AES 加密和解密方法,例如:
```
import java.security.Key;
import java.security.Security;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
public class AESUtil {
private static final String KEY_ALGORITHM = "AES";
private static final String CIPHER_ALGORITHM = "AES/ECB/PKCS7Padding";
static {
Security.addProvider(new BouncyCastleProvider());
}
public static String encrypt(String data, String key) throws Exception {
Key k = new SecretKeySpec(key.getBytes(), KEY_ALGORITHM);
Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM, "BC");
cipher.init(Cipher.ENCRYPT_MODE, k);
byte[] encryptedData = cipher.doFinal(data.getBytes());
return Base64.encodeBase64String(encryptedData);
}
public static String decrypt(String encryptedData, String key) throws Exception {
Key k = new SecretKeySpec(key.getBytes(), KEY_ALGORITHM);
Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM, "BC");
cipher.init(Cipher.DECRYPT_MODE, k);
byte[] decryptedData = cipher.doFinal(Base64.decodeBase64(encryptedData));
return new String(decryptedData);
}
}
```
3. 调用 AESUtil 类进行加密和解密
在 Controller 中引入 AESUtil 类,调用其 encrypt 和 decrypt 方法进行加密和解密操作,例如:
```
import org.springframework.web.bind.annotation.*;
@RestController
public class TestController {
private static final String KEY = "1234567890123456";
@PostMapping("/encrypt")
public String encrypt(@RequestParam String data) throws Exception {
return AESUtil.encrypt(data, KEY);
}
@PostMapping("/decrypt")
public String decrypt(@RequestParam String encryptedData) throws Exception {
return AESUtil.decrypt(encryptedData, KEY);
}
}
```
以上就是在 Spring Boot 中使用 AESUtil 类进行 AES 加密和解密的步骤。
提供的代码不能用 java.security.NoSuchAlgorithmException: Cannot find any provider supporting AES/ECB/ZeroPadding at javax.crypto.Cipher.getInstance(Cipher.java:539) at com.sxd.iec.util.AESUtils.encrypt(AESUtils.java:14) at com.sxd.iec.util.AESUtils.main(AESUtils.java:51)
对不起,我之前提供的代码有误。AES/ECB/ZeroPadding是不被标准Java加密提供程序支持的一个加密算法。在Java标准库中,只支持PKCS5Padding作为填充模式。
以下是修正后的代码示例,使用AES/ECB/PKCS5Padding算法:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class AESUtil {
private static final String ALGORITHM = "AES";
private static final String TRANSFORMATION = "AES/ECB/PKCS5Padding";
public static String encrypt(String plainText, String key) throws Exception {
SecretKeySpec secretKeySpec = new SecretKeySpec(key.getBytes(), ALGORITHM);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec);
byte[] encryptedBytes = cipher.doFinal(plainText.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
public static String decrypt(String encryptedText, String key) throws Exception {
SecretKeySpec secretKeySpec = new SecretKeySpec(key.getBytes(), ALGORITHM);
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec);
byte[] encryptedBytes = Base64.getDecoder().decode(encryptedText);
byte[] decryptedBytes = cipher.doFinal(encryptedBytes);
return new String(decryptedBytes);
}
public static void main(String[] args) {
try {
String plainText = "Hello, World!";
String key = "ThisIsASecretKey"; // 密钥长度必须是16、24或32字节
String encryptedText = encrypt(plainText, key);
System.out.println("Encrypted Text: " + encryptedText);
String decryptedText = decrypt(encryptedText, key);
System.out.println("Decrypted Text: " + decryptedText);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
请注意,此示例使用AES/ECB/PKCS5Padding算法进行加解密,密钥长度必须是16、24或32字节。在示例中,明文为"Hello, World!",密钥为"ThisIsASecretKey"。运行代码后,会输出加密后的密文和解密后的明文。如果仍然出现问题,请确保使用的Java版本支持AES加密算法。
阅读全文
相关推荐
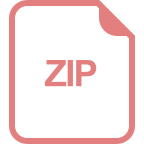
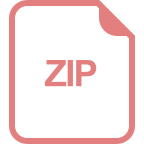
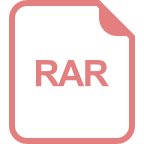
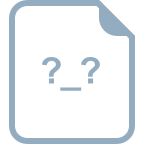
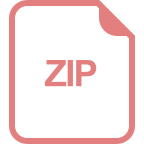
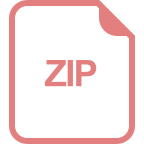
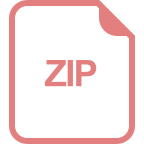
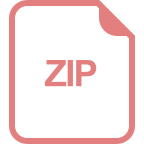
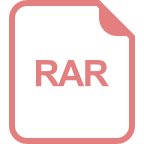