分类分析(CART算法)java实现
时间: 2023-11-10 09:06:43 浏览: 87
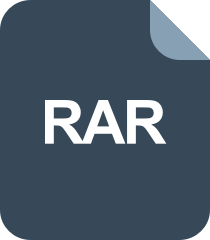
算法分析 (Java版)
CART(Classification and Regression Trees)算法是一种决策树分类算法,适用于离散和连续型特征。下面是一个简单的CART算法的Java实现示例:
```java
import java.util.*;
public class CARTAlgorithm {
private static final int MAX_DEPTH = 10;
private static final int MIN_SIZE = 1;
private static class Node {
private int featureIndex;
private double splitValue;
private Object classification;
private Node left, right;
public Node(int featureIndex, double splitValue, Object classification) {
this.featureIndex = featureIndex;
this.splitValue = splitValue;
this.classification = classification;
left = null;
right = null;
}
public Node(Object classification) {
this.classification = classification;
left = null;
right = null;
}
public boolean isLeaf() {
return left == null && right == null;
}
}
public static Node buildTree(List<double[]> data, Set<Object> classifications) {
return buildTree(data, classifications, 0);
}
private static Node buildTree(List<double[]> data, Set<Object> classifications, int depth) {
if (depth > MAX_DEPTH || data.size() <= MIN_SIZE) {
return new Node(getMostCommonClassification(data, classifications));
}
int featureIndex = 0;
double splitValue = 0;
double lowestMSE = Double.POSITIVE_INFINITY;
List<List<double[]>> splitData = new ArrayList<>();
for (int i = 0; i < data.get(0).length - 1; i++) {
List<double[]> sortedData = new ArrayList<>(data);
sortedData.sort(Comparator.comparingDouble(a -> a[i]));
for (int j = 1; j < sortedData.size(); j++) {
List<double[]> leftData = sortedData.subList(0, j);
List<double[]> rightData = sortedData.subList(j, sortedData.size());
double mse = getMSE(leftData, rightData);
if (mse < lowestMSE) {
featureIndex = i;
splitValue = (sortedData.get(j - 1)[i] + sortedData.get(j)[i]) / 2.0;
lowestMSE = mse;
splitData.clear();
splitData.add(leftData);
splitData.add(rightData);
}
}
}
Node node = new Node(featureIndex, splitValue, null);
node.left = buildTree(splitData.get(0), classifications, depth + 1);
node.right = buildTree(splitData.get(1), classifications, depth + 1);
return node;
}
private static double getMSE(List<double[]> leftData, List<double[]> rightData) {
Object leftClassification = getMostCommonClassification(leftData, new HashSet<>());
Object rightClassification = getMostCommonClassification(rightData, new HashSet<>());
double leftMSE = 0;
double rightMSE = 0;
for (double[] data : leftData) {
leftMSE += Math.pow(data[data.length - 1] - (double) leftClassification, 2);
}
for (double[] data : rightData) {
rightMSE += Math.pow(data[data.length - 1] - (double) rightClassification, 2);
}
return leftMSE + rightMSE;
}
private static Object getMostCommonClassification(List<double[]> data, Set<Object> classifications) {
Map<Object, Integer> counts = new HashMap<>();
for (double[] datum : data) {
Object classification = datum[datum.length - 1];
if (!classifications.contains(classification)) {
classifications.add(classification);
}
counts.put(classification, counts.getOrDefault(classification, 0) + 1);
}
Object mostCommonClassification = null;
int highestCount = Integer.MIN_VALUE;
for (Map.Entry<Object, Integer> entry : counts.entrySet()) {
if (entry.getValue() > highestCount) {
mostCommonClassification = entry.getKey();
highestCount = entry.getValue();
}
}
return mostCommonClassification;
}
public static Object predict(Node root, double[] data) {
while (!root.isLeaf()) {
if (data[root.featureIndex] < root.splitValue) {
root = root.left;
} else {
root = root.right;
}
}
return root.classification;
}
}
```
在这个示例中,我们首先定义了一个节点类,用于表示决策树中的节点。节点可以是叶节点,也可以是内部节点。内部节点包括一个特征索引和一个分割值,用于将数据划分成左右子树。叶节点包括一个分类值。
然后我们定义了一个`buildTree`方法,用于构建决策树。该方法采用递归的方式,根据数据集和分类集合构建节点。在每个节点,我们计算每个特征的最佳分割点,并为其创建一个内部节点。然后我们将数据集分成左右子集,继续构建子树。如果树的深度超过了最大深度或者数据集大小小于等于最小大小,则创建一个叶节点并返回。
我们还定义了一个`predict`方法,用于根据构建的决策树预测新的数据。该方法遍历树并返回叶节点的分类值。
最后,我们使用一些辅助方法来计算节点的最佳分割点、最常见的分类值等。这些方法包括计算均方误差(MSE)、计算出现次数最多的分类值等。
请注意,这只是一个简单的CART算法的示例。实际上,CART算法的实现要复杂得多,并且需要考虑许多细节,例如如何处理缺失值、如何处理连续型特征等。
阅读全文
相关推荐
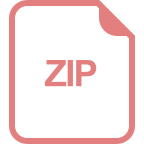

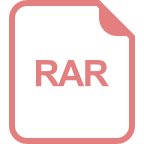
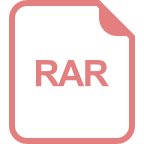
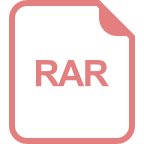
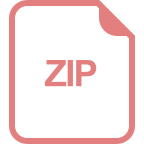
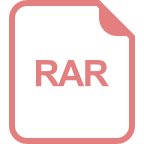
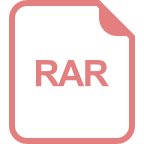


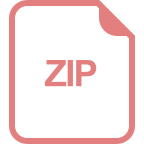
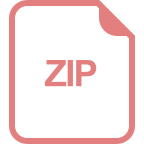
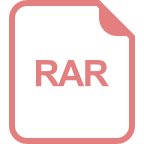
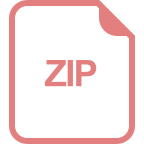
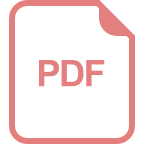
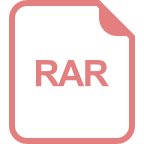
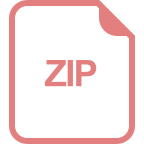