圆角矩形渐变算法 c语言
时间: 2023-10-26 18:20:21 浏览: 179
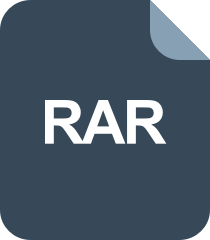
一个简单的圆角矩形算法demo

圆角矩形渐变可以通过以下步骤实现:
1. 画一个填充了渐变色的矩形。
2. 剪切出圆角区域,可以通过使用路径和剪切函数来实现。
3. 在剪切出的圆角区域内使用渐变色填充函数,以实现圆角矩形渐变。
以下是一个简单的圆角矩形渐变算法示例,使用C语言和SDL库:
```c
#include <SDL.h>
void draw_rounded_gradient_rect(SDL_Renderer *renderer, int x, int y, int w, int h, int radius, SDL_Color start_color, SDL_Color end_color) {
// 画一个渐变色矩形
SDL_Rect rect = { x, y, w, h };
SDL_SetRenderDrawBlendMode(renderer, SDL_BLENDMODE_BLEND);
SDL_SetRenderDrawColor(renderer, start_color.r, start_color.g, start_color.b, start_color.a);
SDL_RenderFillRect(renderer, &rect);
SDL_SetRenderDrawColor(renderer, end_color.r, end_color.g, end_color.b, end_color.a);
SDL_RenderDrawRect(renderer, &rect);
// 剪切出圆角区域
SDL_Rect clip_rect = { x, y, w, h };
SDL_RenderSetClipRect(renderer, &clip_rect);
SDL_RenderDrawLine(renderer, x + radius, y, x + w - radius, y);
SDL_RenderDrawLine(renderer, x + radius, y + h, x + w - radius, y + h);
SDL_RenderDrawLine(renderer, x, y + radius, x, y + h - radius);
SDL_RenderDrawLine(renderer, x + w, y + radius, x + w, y + h - radius);
const int diameter = radius * 2;
int cx = x + radius;
int cy = y + radius;
for (int i = 0; i < 4; i++) {
int ax = (i == 1 || i == 2) ? x + w - diameter : x;
int ay = (i == 2 || i == 3) ? y + h - diameter : y;
SDL_RenderDrawLine(renderer, cx, ay, cx, ay + diameter);
SDL_RenderDrawLine(renderer, ax, cy, ax + diameter, cy);
int dx = ax + ((i == 1 || i == 2) ? radius : diameter - radius);
int dy = ay + ((i == 2 || i == 3) ? radius : diameter - radius);
SDL_RenderDrawArc(renderer, dx, dy, radius, i * 90, (i + 1) * 90);
}
// 在圆角区域内使用渐变色填充函数
SDL_SetRenderDrawBlendMode(renderer, SDL_BLENDMODE_BLEND);
SDL_SetRenderDrawColor(renderer, start_color.r, start_color.g, start_color.b, start_color.a);
for (int i = 0; i < radius; i++) {
float t = (float)i / radius;
SDL_Color color = { start_color.r + (end_color.r - start_color.r) * t,
start_color.g + (end_color.g - start_color.g) * t,
start_color.b + (end_color.b - start_color.b) * t,
start_color.a + (end_color.a - start_color.a) * t };
int dx = cx - i;
int dy = cy - i;
int dw = i * 2;
int dh = i * 2;
SDL_Rect gradient_rect = { dx, dy, dw, dh };
SDL_SetRenderDrawColor(renderer, color.r, color.g, color.b, color.a);
SDL_RenderFillRect(renderer, &gradient_rect);
}
}
```
需要注意的是,该算法仅演示了如何实现圆角矩形渐变,如果需要更加复杂的效果,可以根据实际需求进行修改。
阅读全文
相关推荐
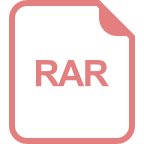
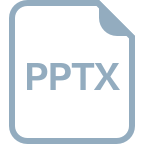
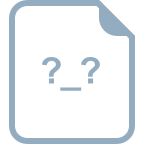
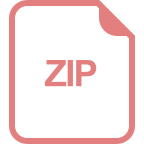
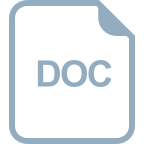
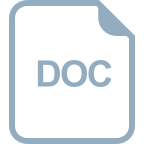

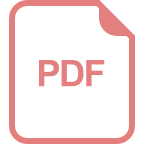
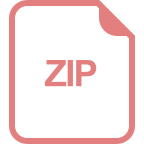
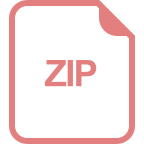
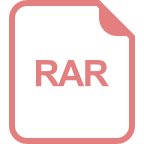
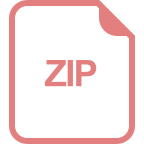
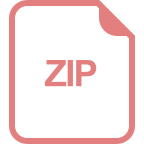
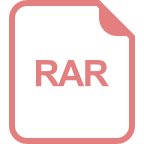
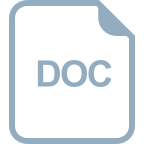