hashmap cannot be cast to
时间: 2023-04-23 09:04:04 浏览: 936
这个错误提示意味着您试图将一个HashMap类型的对象强制转换成另一种类型,但目标类型不是HashMap或其子类,因此转换失败了。
要解决这个问题,您需要检查您的代码并确保所有类型转换都是正确的。如果您确定您的代码没有类型转换问题,则可能是由于您的程序在运行时出现了某种异常或错误,导致对象类型不一致。
如果您需要更具体的帮助,请提供更多上下文和代码示例,以便我能够更好地帮助您解决问题。
相关问题
hashmap cannot be cast to list
The error "hashmap cannot be cast to list" occurs when you try to assign or convert a HashMap object to a List object directly, but they are incompatible types.
HashMap is a key-value pair data structure that implements the Map interface, while List is an ordered collection of elements that allows duplicates and implements the List interface.
To resolve this issue, you need to convert the HashMap into a List by extracting the values or keys from the HashMap and adding them to the List. Here's an example:
```java
import java.util.*;
public class Example {
public static void main(String[] args) {
HashMap<Integer, String> hashMap = new HashMap<>();
hashMap.put(1, "One");
hashMap.put(2, "Two");
hashMap.put(3, "Three");
List<String> list = new ArrayList<>(hashMap.values()); // OR
// List<Integer> list = new ArrayList<>(hashMap.keySet());
System.out.println(list);
}
}
```
In this example, we extract the values from the HashMap using the `values()` method and create a new ArrayList with those values. Alternatively, you can use `keySet()` method to extract the keys and add them to the List.
Make sure to choose the appropriate data type for your List based on the values or keys you want to extract from the HashMap.
java.util.HashMap cannot be cast to
"java.util.HashMap cannot be cast to"是一个Java中的异常信息,表示无法将HashMap类型转换为其他类型。
在Java中,类型转换是一种将一个对象从一种类型转换为另一种类型的操作。但是,如果对象的实际类型与要转换的类型不兼容,就会抛出ClassCastException异常。
在这个具体的异常信息中,表示尝试将一个HashMap对象转换为其他类型,但是由于HashMap与目标类型不兼容,所以抛出了该异常。
要解决这个问题,你可以检查代码中的类型转换部分,确保将HashMap对象转换为正确的类型。或者,你可以使用instanceof关键字来检查对象的类型,然后再进行相应的操作。
相关推荐
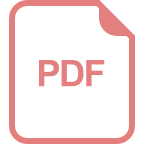
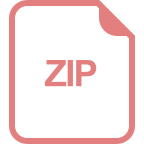












