qt通过QGraphics实现箭头指向动画效果
时间: 2023-10-15 15:04:41 浏览: 78
您可以通过QGraphicsItemAnimation类来实现箭头指向动画效果。具体实现步骤如下:
1. 创建箭头图形项,并将其添加到场景中。
2. 创建QGraphicsItemAnimation对象,并将箭头图形项设置为其目标项。
3. 创建QPropertyAnimation对象,并将其设置为QGraphicsItemAnimation的动画属性。
4. 设置QPropertyAnimation对象的起始值和结束值,以实现箭头指向动画效果。
5. 将QPropertyAnimation对象添加到QGraphicsItemAnimation对象中。
6. 启动QGraphicsItemAnimation对象的动画。
以下是一个简单的示例代码,可以实现箭头指向动画效果:
```python
from PyQt5.QtWidgets import *
from PyQt5.QtCore import *
from PyQt5.QtGui import *
class ArrowItem(QGraphicsPathItem):
def __init__(self, parent=None):
super().__init__(parent)
self.setFlag(QGraphicsItem.ItemIsMovable)
path = QPainterPath()
path.moveTo(0, 0)
path.lineTo(30, 0)
path.lineTo(25, -5)
path.moveTo(30, 0)
path.lineTo(25, 5)
self.setPath(path)
class ArrowAnimation(QGraphicsItemAnimation):
def __init__(self, arrowItem, parent=None):
super().__init__(parent)
self.setItem(arrowItem)
self.anim = QPropertyAnimation(self, b"rotation")
self.anim.setEasingCurve(QEasingCurve.OutQuad)
self.anim.setDuration(1000)
def setStartAngle(self, angle):
self.anim.setStartValue(angle)
def setEndAngle(self, angle):
self.anim.setEndValue(angle)
def start(self):
self.anim.start()
if __name__ == "__main__":
app = QApplication([])
view = QGraphicsView()
scene = QGraphicsScene()
arrow = ArrowItem()
scene.addItem(arrow)
arrowAnim = ArrowAnimation(arrow)
arrowAnim.setStartAngle(0)
arrowAnim.setEndAngle(90)
arrowAnim.start()
view.setScene(scene)
view.show()
app.exec_()
```
在这个示例中,我们创建了一个ArrowItem类来表示箭头图形项,它继承自QGraphicsPathItem类。我们还创建了一个ArrowAnimation类来表示箭头指向动画效果,它继承自QGraphicsItemAnimation类。在ArrowAnimation类中,我们创建了一个QPropertyAnimation对象来控制箭头旋转角度的动画效果。我们还实现了setStartAngle()和setEndAngle()方法,用于设置动画的起始角度和结束角度。最后,我们在主函数中创建了箭头图形项和箭头指向动画对象,并添加到场景中。调用start()方法启动动画效果。
相关推荐
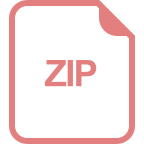
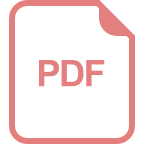














