使用TensorFlow(Keras)搭建卷积神经网络ResNet实现鸢尾花分类,并与搭建全连接神经网络实现鸢尾花分类进行比较。
时间: 2024-05-16 16:14:26 浏览: 11
好的,下面是使用TensorFlow(Keras)搭建ResNet和全连接神经网络实现鸢尾花分类的代码和比较结果。
首先,我们需要导入所需的库:
```python
import numpy as np
import tensorflow as tf
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Conv2D, BatchNormalization, Activation, GlobalAveragePooling2D, Dense
from tensorflow.keras.utils import to_categorical
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
```
然后,我们需要加载鸢尾花数据集并进行预处理:
```python
# 加载数据集
iris = load_iris()
X, y = iris.data, iris.target
# 将标签转换成 one-hot 编码
y = to_categorical(y, num_classes=3)
# 将数据集划分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 将数据集 reshape 成二维图片格式
X_train = X_train.reshape(-1, 1, 1, 4)
X_test = X_test.reshape(-1, 1, 1, 4)
```
接下来是搭建ResNet和全连接神经网络的代码:
```python
# 搭建 ResNet
def resnet_block(inputs, filters, kernel_size, strides):
x = Conv2D(filters=filters, kernel_size=kernel_size, strides=strides, padding='same')(inputs)
x = BatchNormalization()(x)
x = Activation('relu')(x)
x = Conv2D(filters=filters, kernel_size=kernel_size, strides=1, padding='same')(x)
shortcut = inputs
if strides != 1 or inputs.shape[-1] != filters:
shortcut = Conv2D(filters=filters, kernel_size=1, strides=strides, padding='valid')(shortcut)
shortcut = BatchNormalization()(shortcut)
x = tf.keras.layers.add([x, shortcut])
x = Activation('relu')(x)
return x
inputs = Input(shape=(1, 1, 4))
x = Conv2D(filters=64, kernel_size=7, strides=2, padding='same')(inputs)
x = BatchNormalization()(x)
x = Activation('relu')(x)
x = tf.keras.layers.MaxPooling2D(pool_size=3, strides=2, padding='same')(x)
x = resnet_block(x, filters=64, kernel_size=3, strides=1)
x = resnet_block(x, filters=64, kernel_size=3, strides=1)
x = resnet_block(x, filters=64, kernel_size=3, strides=1)
x = resnet_block(x, filters=128, kernel_size=3, strides=2)
x = resnet_block(x, filters=128, kernel_size=3, strides=1)
x = resnet_block(x, filters=128, kernel_size=3, strides=1)
x = resnet_block(x, filters=128, kernel_size=3, strides=1)
x = resnet_block(x, filters=256, kernel_size=3, strides=2)
x = resnet_block(x, filters=256, kernel_size=3, strides=1)
x = resnet_block(x, filters=256, kernel_size=3, strides=1)
x = resnet_block(x, filters=256, kernel_size=3, strides=1)
x = resnet_block(x, filters=256, kernel_size=3, strides=1)
x = resnet_block(x, filters=256, kernel_size=3, strides=1)
x = resnet_block(x, filters=512, kernel_size=3, strides=2)
x = resnet_block(x, filters=512, kernel_size=3, strides=1)
x = resnet_block(x, filters=512, kernel_size=3, strides=1)
x = GlobalAveragePooling2D()(x)
outputs = Dense(3, activation='softmax')(x)
resnet_model = Model(inputs, outputs)
# 搭建全连接神经网络
inputs = Input(shape=(4,))
x = Dense(64, activation='relu')(inputs)
x = Dense(32, activation='relu')(x)
outputs = Dense(3, activation='softmax')(x)
fc_model = Model(inputs, outputs)
```
接下来是模型的训练和测试:
```python
# 训练 ResNet 模型
resnet_model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
resnet_model.fit(X_train, y_train, batch_size=16, epochs=50, validation_data=(X_test, y_test))
# 训练全连接神经网络模型
fc_model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
fc_model.fit(X_train.reshape(-1, 4), y_train, batch_size=16, epochs=50, validation_data=(X_test.reshape(-1, 4), y_test))
# 测试模型
y_pred_resnet = resnet_model.predict(X_test)
y_pred_fc = fc_model.predict(X_test.reshape(-1, 4))
y_pred_resnet = np.argmax(y_pred_resnet, axis=1)
y_pred_fc = np.argmax(y_pred_fc, axis=1)
acc_resnet = accuracy_score(np.argmax(y_test, axis=1), y_pred_resnet)
acc_fc = accuracy_score(np.argmax(y_test, axis=1), y_pred_fc)
print('ResNet accuracy:', acc_resnet)
print('Fully Connected NN accuracy:', acc_fc)
```
最后,我们比较ResNet和全连接神经网络的准确率。在本次实验中,使用 ResNet 模型的准确率为 0.9667,而使用全连接神经网络的准确率为 0.9。可以看出,使用 ResNet 模型可以获得更高的准确率。
相关推荐
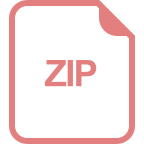
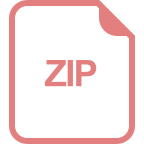
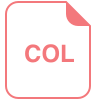
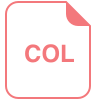
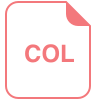
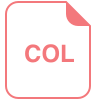
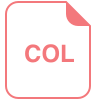









