写一个C++程序,在界面要求输入顾客的信息,然后保存到文件,然后可以在运行界面显示货物流量和消费人群的统计
时间: 2024-04-30 15:21:19 浏览: 26
以下是一个简单的 C++ 程序,用于输入顾客信息并将其保存到文件中。该程序还会统计货物流量和消费人群的统计,并在运行界面显示。
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <map>
using namespace std;
struct Customer {
string name;
int age;
string gender;
string product;
double price;
};
void saveToFile(const vector<Customer>& customers, const string& filename) {
ofstream outFile(filename);
if (!outFile) {
cerr << "Error: cannot open file " << filename << endl;
exit(1);
}
for (const auto& customer : customers) {
outFile << customer.name << ',' << customer.age << ',' << customer.gender << ',' << customer.product << ',' << customer.price << endl;
}
outFile.close();
}
void readFromFile(vector<Customer>& customers, const string& filename) {
ifstream inFile(filename);
if (!inFile) {
cerr << "Error: cannot open file " << filename << endl;
exit(1);
}
string line;
while (getline(inFile, line)) {
Customer customer;
size_t pos = line.find(',');
customer.name = line.substr(0, pos);
line = line.substr(pos + 1);
pos = line.find(',');
customer.age = stoi(line.substr(0, pos));
line = line.substr(pos + 1);
pos = line.find(',');
customer.gender = line.substr(0, pos);
line = line.substr(pos + 1);
pos = line.find(',');
customer.product = line.substr(0, pos);
line = line.substr(pos + 1);
customer.price = stod(line);
customers.push_back(customer);
}
inFile.close();
}
void printStatistics(const vector<Customer>& customers) {
map<string, int> productCount;
map<string, int> genderCount;
int totalRevenue = 0;
for (const auto& customer : customers) {
productCount[customer.product]++;
genderCount[customer.gender]++;
totalRevenue += customer.price;
}
cout << "Product statistics:" << endl;
for (const auto& p : productCount) {
cout << p.first << ": " << p.second << " customers" << endl;
}
cout << "Gender statistics:" << endl;
for (const auto& g : genderCount) {
cout << g.first << ": " << g.second << " customers" << endl;
}
cout << "Total revenue: $" << totalRevenue << endl;
}
int main() {
vector<Customer> customers;
string filename = "customers.txt";
// Read from file
readFromFile(customers, filename);
// Input new customer information
cout << "Enter customer information (name, age, gender, product, price):" << endl;
Customer customer;
getline(cin, customer.name, ',');
cin >> customer.age;
cin.ignore();
getline(cin, customer.gender, ',');
getline(cin, customer.product, ',');
cin >> customer.price;
customers.push_back(customer);
// Save to file
saveToFile(customers, filename);
// Print statistics
printStatistics(customers);
return 0;
}
```
在该程序中,我们定义了一个 `Customer` 结构体,用于存储顾客的信息。`saveToFile` 和 `readFromFile` 函数分别用于将顾客信息保存到文件和从文件中读取顾客信息。`printStatistics` 函数用于统计货物流量和消费人群的统计,并在运行界面显示。
在程序运行时,我们首先从文件中读取已有的顾客信息。然后,我们要求用户输入新的顾客信息,并将其添加到 `customers` 向量中。接着,我们将 `customers` 向量中的所有顾客信息保存到文件中。最后,我们打印货物流量和消费人群的统计信息。
相关推荐
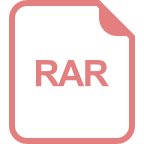
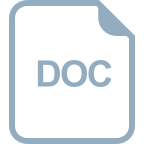
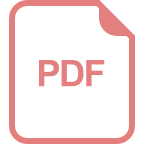














