参考教材中的生产者消费者算法,创建5个进程,其中两个进程为生产者进程,3个进程为
时间: 2023-08-08 15:05:47 浏览: 167
消费者进程。生产者进程每次生产一个随机数并将其放入缓冲区中,消费者进程从缓冲区中取出随机数并输出。如果缓冲区已满,则生产者进程需要等待;如果缓冲区为空,则消费者进程需要等待。
下面是该算法的实现:
```python
import threading
import time
import random
BUFFER_SIZE = 10
buffer = []
mutex = threading.Lock()
not_full = threading.Condition(mutex)
not_empty = threading.Condition(mutex)
class Producer(threading.Thread):
def run(self):
while True:
not_full.acquire()
if len(buffer) == BUFFER_SIZE:
print("Buffer is full, producer is waiting")
not_full.wait()
num = random.randint(1, 100)
buffer.append(num)
print("Produced:", num)
not_empty.notify()
not_full.release()
time.sleep(random.random())
class Consumer(threading.Thread):
def run(self):
while True:
not_empty.acquire()
if not buffer:
print("Buffer is empty, consumer is waiting")
not_empty.wait()
num = buffer.pop(0)
print("Consumed:", num)
not_full.notify()
not_empty.release()
time.sleep(random.random())
if __name__ == "__main__":
for i in range(2):
p = Producer()
p.start()
for i in range(3):
c = Consumer()
c.start()
```
在这个实现中,使用了 Python 的 `threading` 模块创建了生产者和消费者线程。`BUFFER_SIZE` 定义了缓冲区的大小,`buffer` 是缓冲区。`mutex` 是互斥锁,用来保证在修改缓冲区时的互斥性。`not_full` 和 `not_empty` 分别是条件变量,用来实现生产者和消费者线程之间的同步。
生产者线程 `Producer` 每次随机生成一个整数,并将其加入缓冲区中。如果缓冲区已满,则等待条件变量 `not_full`。如果缓冲区未满,则加入数据并唤醒条件变量 `not_empty`。最后睡眠一段时间。
消费者线程 `Consumer` 每次从缓冲区中取出一个整数并输出。如果缓冲区为空,则等待条件变量 `not_empty`。如果缓冲区不为空,则取出数据并唤醒条件变量 `not_full`。最后睡眠一段时间。
在主函数中,创建了 2 个生产者线程和 3 个消费者线程,并启动它们。
阅读全文
相关推荐



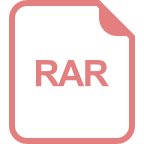
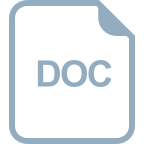
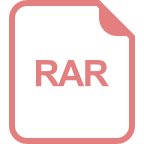
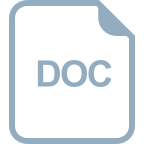
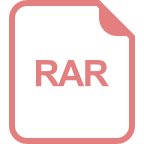
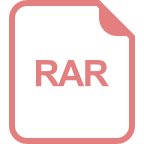
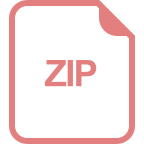
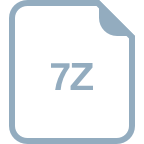
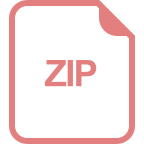