使用DS18B20测温,将测温结果显示在LCD1602中
时间: 2024-02-21 20:58:34 浏览: 44
好的,以下是一个使用DS18B20测温,并将测温结果显示在LCD1602中的示例代码:
```python
import os
import glob
import time
import smbus
# 定义DS18B20的设备文件路径
device_file = '/sys/bus/w1/devices/28-*/w1_slave'
# 初始化LCD1602
def lcd_init():
# 打开I2C总线
bus = smbus.SMBus(1)
# 设置LCD的地址
LCD_ADDR = 0x27
# 初始化LCD
bus.write_byte_data(LCD_ADDR, 0x00, 0x38)
bus.write_byte_data(LCD_ADDR, 0x00, 0x39)
bus.write_byte_data(LCD_ADDR, 0x00, 0x14)
bus.write_byte_data(LCD_ADDR, 0x00, 0x70)
bus.write_byte_data(LCD_ADDR, 0x00, 0x56)
bus.write_byte_data(LCD_ADDR, 0x00, 0x6c)
time.sleep(0.2)
bus.write_byte_data(LCD_ADDR, 0x00, 0x38)
bus.write_byte_data(LCD_ADDR, 0x00, 0x0c)
bus.write_byte_data(LCD_ADDR, 0x00, 0x01)
time.sleep(0.2)
return bus, LCD_ADDR
# 在LCD上显示温度
def lcd_show_temperature(bus, LCD_ADDR, temperature):
# 清空LCD屏幕
bus.write_byte_data(LCD_ADDR, 0x00, 0x01)
time.sleep(0.2)
# 将温度转化为字符串
temperature_str = '{:.2f}'.format(temperature)
# 在LCD上显示温度
bus.write_byte_data(LCD_ADDR, 0x40, ord('T'))
bus.write_byte_data(LCD_ADDR, 0x40+1, ord('e'))
bus.write_byte_data(LCD_ADDR, 0x40+2, ord('m'))
bus.write_byte_data(LCD_ADDR, 0x40+3, ord('p'))
bus.write_byte_data(LCD_ADDR, 0x40+4, ord(':'))
for i, c in enumerate(temperature_str):
bus.write_byte_data(LCD_ADDR, 0x40+5+i, ord(c))
# 读取温度数据
def read_temperature():
# 找到DS18B20的设备文件路径
devicelist = glob.glob(device_file)
if not devicelist:
return None
device_file = devicelist[0]
# 读取设备文件中的温度数据
with open(device_file, 'r') as f:
lines = f.readlines()
# 解析温度数据
if lines[0].strip()[-3:] != 'YES':
return None
temperature = lines[1].find('t=')
if temperature == -1:
return None
temperature = float(lines[1][temperature+2:]) / 1000.0
return temperature
# 主函数
def main():
# 初始化LCD
bus, LCD_ADDR = lcd_init()
while True:
# 读取温度
temperature = read_temperature()
if temperature is not None:
# 在LCD上显示温度
lcd_show_temperature(bus, LCD_ADDR, temperature)
# 等待1秒钟
time.sleep(1)
if __name__ == '__main__':
main()
```
这个程序首先会初始化LCD1602,并进入一个无限循环中。在每次循环中,程序会读取DS18B20的温度,并将温度值显示在LCD1602的第二行上。注意,这个程序需要使用SMBus库来控制I2C总线。另外,这个程序只在温度读取成功时才会更新LCD上的温度值。
阅读全文
相关推荐
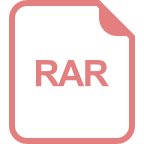
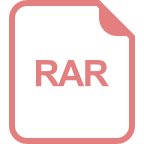
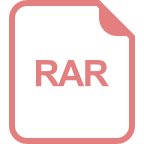
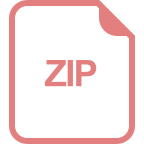
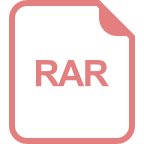
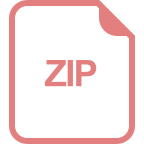
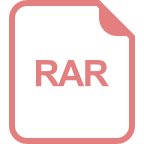
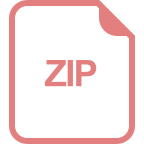
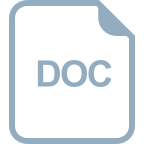
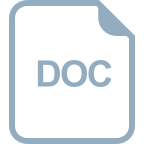


