springboot配置pgsql数据源,完成单表数据查询
时间: 2023-09-10 12:04:45 浏览: 84
### 回答1:
在Spring Boot应用程序中配置PostgreSQL数据源,需要在应用程序的application.properties文件中添加以下属性:spring.datasource.url= jdbc:postgresql://<host>:<port>/<database>spring.datasource.username= <username>spring.datasource.password= <password>spring.datasource.driver-class-name=org.postgresql.Driver然后,要完成单表数据查询,可以使用Spring Data JPA的Repository接口,它提供了一组简单的方法,用于查询单个实体或实体列表。
### 回答2:
Spring Boot是一个用于开发Java应用程序的框架,它简化了配置和部署的过程。下面是使用Spring Boot配置和使用PostgreSQL数据源完成单表数据查询的步骤:
1. 首先,确保已经在Maven或Gradle配置文件中添加了Spring Boot和PostgreSQL的依赖。
2. 在application.properties文件中添加以下数据库配置信息:
```
spring.datasource.url=jdbc:postgresql://localhost:5432/your-database-name
spring.datasource.username=your-username
spring.datasource.password=your-password
spring.datasource.driver-class-name=org.postgresql.Driver
```
将`your-database-name`替换为你的数据库名称,`your-username`和`your-password`替换为你的登录凭证。
3. 创建一个实体类,用于映射数据库中的表。例如,如果有一个名为`User`的表,可以创建以下Java类:
```java
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// 省略构造方法、getter和setter等
}
```
这里使用了JPA的注解来定义实体类和表之间的映射关系。
4. 创建一个继承自`JpaRepository`接口的数据访问层接口,用于执行数据库查询操作。例如,对于上述的`User`实体类,可以创建以下接口:
```java
public interface UserRepository extends JpaRepository<User, Long> {
// 定义需要的查询方法
}
```
5. 在业务逻辑层或控制器中使用`UserRepository`接口执行数据库查询操作。例如,以下代码演示了如何查询所有用户:
```java
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public List<User> getAllUsers() {
return userRepository.findAll();
}
}
```
通过`userRepository.findAll()`方法可以获取所有的用户记录。
6. 最后,在控制器类中使用`UserService`进行用户操作的调用。例如,以下代码演示了如何使用`UserService`查询所有用户,并返回JSON格式的数据:
```java
@RestController
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/users")
public List<User> getAllUsers() {
return userService.getAllUsers();
}
}
```
进行请求`GET /users`将会返回所有用户的JSON数据。
以上就是使用Spring Boot配置和使用PostgreSQL数据源完成单表数据查询的基本步骤。根据实际需求,你可以在`UserRepository`接口中定义更多的查询方法和`UserService`中编写更多的业务逻辑。
### 回答3:
要在Spring Boot中配置PostgreSQL数据源并完成单表数据查询,可以按照以下步骤进行操作:
1. 在pom.xml文件中添加相关依赖:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
</dependency>
</dependencies>
```
2. 在application.properties文件中配置数据库连接信息:
```properties
spring.datasource.url=jdbc:postgresql://localhost:5432/database_name
spring.datasource.username=username
spring.datasource.password=password
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.PostgreSQLDialect
spring.jpa.hibernate.ddl-auto=update
```
其中,`database_name`是数据库名称,`username`和`password`是数据库的用户名和密码。`hibernate.dialect`指定了使用的Hibernate方言。
3. 创建实体类,例如名为`User`的用户类:
```java
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username")
private String username;
// 其他属性和方法省略
}
```
4. 创建Repository接口来管理实体类,例如名为`UserRepository`的用户仓库接口:
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
// 可以根据需要添加其他自定义方法
}
```
5. 在需要查询数据的地方使用注入的Repository接口进行数据查询:
```java
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> getAllUsers() {
return userRepository.findAll();
}
}
```
以上是配置并完成单表数据查询的基本步骤,你可以根据具体需求进行进一步的操作和扩展。
相关推荐
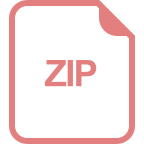
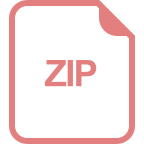














