2. 定义一个既能接收任意多个位置参数,又能接收任意多个关键字参数的函数myfun,至少采用两种不同长度的实参进行调用,并通过myfun将实参内容打印出来。
时间: 2024-05-01 14:23:53 浏览: 14
可以使用*args和**kwargs参数来实现这个功能,*args表示任意多个位置参数,**kwargs表示任意多个关键字参数。下面是一个例子:
```python
def myfun(*args, **kwargs):
print("位置参数:")
for arg in args:
print(arg)
print("关键字参数:")
for key, value in kwargs.items():
print("{} = {}".format(key, value))
# 调用myfun函数
myfun(1, 2, 3, a='apple', b='banana')
myfun('hello', 'world', name='Alice', age=18)
```
输出结果如下:
```
位置参数:
1
2
3
关键字参数:
a = apple
b = banana
位置参数:
hello
world
关键字参数:
name = Alice
age = 18
```
第一次调用传入了3个位置参数和2个关键字参数,第二次调用传入了2个位置参数和2个关键字参数。在myfun函数内部,使用for循环遍历args和kwargs,将它们的值依次打印出来。
相关问题
定义一个既能接收任意多个位置参数,又能接收任意多个关键字参数的函数myfun,至少采用两种不同长度的实参进行调用,并通过myfun将实参内容打印出来。
可以使用以下代码来定义一个既能接收任意多个位置参数,又能接收任意多个关键字参数的函数myfun,并通过print语句将实参内容打印出来:
```python
def myfun(*args, **kwargs):
print("位置参数:", args)
print("关键字参数:", kwargs)
# 调用myfun函数,传入两个位置参数和两个关键字参数
myfun(1, 2, name='John', age=25)
# 调用myfun函数,传入三个位置参数和三个关键字参数
myfun(3, 4, 5, name='Jane', age=30, city='New York')
```
输出结果为:
```
位置参数: (1, 2)
关键字参数: {'name': 'John', 'age': 25}
位置参数: (3, 4, 5)
关键字参数: {'name': 'Jane', 'age': 30, 'city': 'New York'}
```
在这个例子中,`*args`用来接收任意多个位置参数,`**kwargs`用来接收任意多个关键字参数。函数体内,我们使用了print语句将实参内容打印出来。在第一个调用中,我们传入了两个位置参数和两个关键字参数,而在第二个调用中,我们传入了三个位置参数和三个关键字参数。
python定义一个函数myfun返回多个对象,并将函数结果打印出来
可以使用元组(tuple)来返回多个对象,以下是一个例子:
```python
def myfun():
a = 1
b = 2
c = 3
return a, b, c
result = myfun()
print(result)
```
输出结果为:`(1, 2, 3)`,其中,`result` 是一个元组,包含了函数 `myfun` 返回的三个值。你可以通过下标来访问每一个值,例如 `result[0]` 返回 `1`,`result[1]` 返回 `2`。
相关推荐
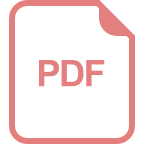












