简单的 Vue 腾讯地址检索对话框组件的实现
时间: 2024-06-09 13:07:20 浏览: 8
首先,你需要在 Vue 中安装 Tencent 地图的 JavaScript API,并在 `index.html` 文件中引入该 API:
```html
<script src="https://map.qq.com/api/js?v=2.exp&key=YOUR_KEY"></script>
```
其中 `YOUR_KEY` 是你在腾讯云申请的 API Key。
然后,你可以创建一个 `MapDialog` 组件,该组件包含一个按钮,点击该按钮会弹出一个对话框,让用户输入地址并在地图上显示该地址。
```vue
<template>
<div>
<button @click="showDialog">检索地址</button>
<div v-if="visible">
<div ref="map" style="width: 600px; height: 400px;"></div>
<input v-model="address" placeholder="请输入地址">
<button @click="search">搜索</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
visible: false,
address: "",
map: null,
marker: null
};
},
methods: {
showDialog() {
this.visible = true;
this.initMap();
},
initMap() {
this.map = new qq.maps.Map(this.$refs.map, {
center: new qq.maps.LatLng(39.916527, 116.397128),
zoom: 13
});
},
search() {
const geocoder = new qq.maps.Geocoder();
geocoder.getLocation(this.address, (result) => {
if (result && result.detail && result.detail.location) {
const location = result.detail.location;
if (!this.marker) {
this.marker = new qq.maps.Marker({
position: location,
map: this.map
});
} else {
this.marker.setPosition(location);
}
this.map.setCenter(location);
} else {
alert("地址不存在");
}
});
}
}
};
</script>
```
在该组件中,我们先定义了一些数据,包括对话框的可见性、输入的地址、地图对象和标记对象。在 `showDialog` 方法中,我们将对话框的可见性设置为 `true`,并调用 `initMap` 方法初始化地图。在 `initMap` 方法中,我们使用腾讯地图 API 创建了一个地图对象,并将其显示在页面上。在 `search` 方法中,我们使用腾讯地图 API 的 `Geocoder` 类来检索输入的地址,并在地图上显示该地址的位置。
最后,在父组件中引用 `MapDialog` 组件即可:
```vue
<template>
<div>
<map-dialog></map-dialog>
</div>
</template>
<script>
import MapDialog from "./MapDialog.vue";
export default {
components: {
MapDialog
}
};
</script>
```
相关推荐
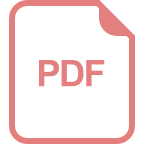
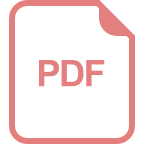














