java aes加密登录账号
时间: 2023-11-18 20:48:49 浏览: 162
以下是一个Java AES加密登录账号的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.security.MessageDigest;
import java.util.Base64;
public class AesEncryption {
private static final String SECRET_KEY = "mysecretkey12345";
private static final String INIT_VECTOR = "encryptionIntVec";
public static String encrypt(String value) throws Exception {
IvParameterSpec iv = new IvParameterSpec(INIT_VECTOR.getBytes("UTF-8"));
SecretKeySpec keySpec = new SecretKeySpec(SECRET_KEY.getBytes("UTF-8"), "AES");
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5PADDING");
cipher.init(Cipher.ENCRYPT_MODE, keySpec, iv);
byte[] encrypted = cipher.doFinal(value.getBytes());
return Base64.getEncoder().encodeToString(encrypted);
}
public static String decrypt(String encrypted) throws Exception {
IvParameterSpec iv = new IvParameterSpec(INIT_VECTOR.getBytes("UTF-8"));
SecretKeySpec keySpec = new SecretKeySpec(SECRET_KEY.getBytes("UTF-8"), "AES");
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5PADDING");
cipher.init(Cipher.DECRYPT_MODE, keySpec, iv);
byte[] original = cipher.doFinal(Base64.getDecoder().decode(encrypted));
return new String(original);
}
public static void main(String[] args) throws Exception {
String username = "myusername";
String password = "mypassword";
String encryptedUsername = encrypt(username);
String encryptedPassword = encrypt(password);
System.out.println("Encrypted username: " + encryptedUsername);
System.out.println("Encrypted password: " + encryptedPassword);
// Simulating login process
String inputUsername = "myusername";
String inputPassword = "mypassword";
String hashedUsername = encrypt(inputUsername);
String hashedPassword = encrypt(inputPassword);
if (hashedUsername.equals(encryptedUsername) && hashedPassword.equals(encryptedPassword)) {
System.out.println("Login successful!");
} else {
System.out.println("Login failed!");
}
}
}
```
该示例使用AES/CBC/PKCS5PADDING加密算法进行加密和解密,并使用Base64编码将加密后的字节数组转换为字符串。在主方法中,我们首先使用encrypt方法对用户名和密码进行加密,并将加密后的值存储在变量encryptedUsername和encryptedPassword中。然后,我们模拟了登录过程,将输入的用户名和密码进行加密并与存储的加密值进行比较。如果它们匹配,我们将输出“登录成功!”否则,我们将输出“登录失败!”。
阅读全文
相关推荐


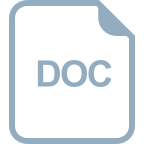
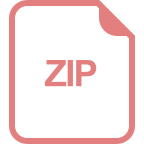
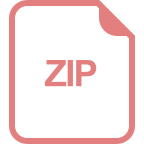
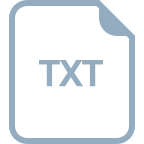
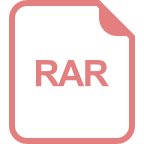
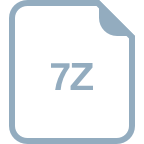
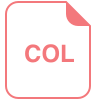
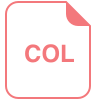

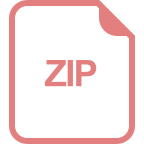
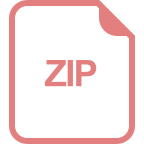
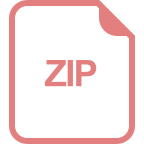
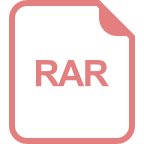
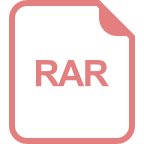