Java简单增删改查项目
时间: 2023-07-11 20:16:33 浏览: 44
好的,我可以为您提供一个简单的 Java 增删改查项目示例。
首先,您需要创建一个 Java 项目,并创建一个名为 "Employee" 的类,表示雇员对象,代码如下:
```java
public class Employee {
private int id;
private String name;
private int age;
private String position;
public Employee(int id, String name, int age, String position) {
this.id = id;
this.name = name;
this.age = age;
this.position = position;
}
// getter and setter methods
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getPosition() {
return position;
}
public void setPosition(String position) {
this.position = position;
}
}
```
接下来,您需要创建一个数据库表来存储雇员信息。假设您创建了一个名为 "employee" 的表,包含以下列:
- id INT PRIMARY KEY
- name VARCHAR(50)
- age INT
- position VARCHAR(50)
然后,您需要创建一个名为 "EmployeeDAO" 的类,用于执行增删改查操作。代码如下:
```java
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class EmployeeDAO {
private Connection connection;
public EmployeeDAO() {
try {
// 连接数据库
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "root", "password");
} catch (SQLException e) {
e.printStackTrace();
}
}
// 查询所有雇员
public List<Employee> findAll() {
List<Employee> employees = new ArrayList<>();
try {
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM employee");
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
int age = resultSet.getInt("age");
String position = resultSet.getString("position");
employees.add(new Employee(id, name, age, position));
}
} catch (SQLException e) {
e.printStackTrace();
}
return employees;
}
// 根据 ID 查询雇员
public Employee findById(int id) {
Employee employee = null;
try {
PreparedStatement preparedStatement = connection.prepareStatement("SELECT * FROM employee WHERE id = ?");
preparedStatement.setInt(1, id);
ResultSet resultSet = preparedStatement.executeQuery();
if (resultSet.next()) {
String name = resultSet.getString("name");
int age = resultSet.getInt("age");
String position = resultSet.getString("position");
employee = new Employee(id, name, age, position);
}
} catch (SQLException e) {
e.printStackTrace();
}
return employee;
}
// 添加雇员
public void addEmployee(Employee employee) {
try {
PreparedStatement preparedStatement = connection.prepareStatement("INSERT INTO employee(id, name, age, position) VALUES (?, ?, ?, ?)");
preparedStatement.setInt(1, employee.getId());
preparedStatement.setString(2, employee.getName());
preparedStatement.setInt(3, employee.getAge());
preparedStatement.setString(4, employee.getPosition());
preparedStatement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 更新雇员信息
public void updateEmployee(Employee employee) {
try {
PreparedStatement preparedStatement = connection.prepareStatement("UPDATE employee SET name = ?, age = ?, position = ? WHERE id = ?");
preparedStatement.setString(1, employee.getName());
preparedStatement.setInt(2, employee.getAge());
preparedStatement.setString(3, employee.getPosition());
preparedStatement.setInt(4, employee.getId());
preparedStatement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 删除雇员
public void deleteEmployee(int id) {
try {
PreparedStatement preparedStatement = connection.prepareStatement("DELETE FROM employee WHERE id = ?");
preparedStatement.setInt(1, id);
preparedStatement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
最后,您可以在主方法中测试这些操作,例如:
```java
public static void main(String[] args) {
EmployeeDAO dao = new EmployeeDAO();
// 查询所有雇员
List<Employee> employees = dao.findAll();
for (Employee employee : employees) {
System.out.println(employee.getName());
}
// 根据 ID 查询雇员
Employee employee = dao.findById(1);
System.out.println(employee.getName());
// 添加雇员
Employee newEmployee = new Employee(4, "Tom", 25, "Developer");
dao.addEmployee(newEmployee);
// 更新雇员信息
employee.setName("John");
employee.setAge(30);
dao.updateEmployee(employee);
// 删除雇员
dao.deleteEmployee(4);
}
```
希望这个示例能帮助您理解 Java 的增删改查操作。
相关推荐
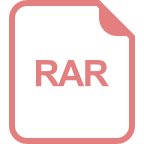














