用c++实现csmaca协议
时间: 2023-08-24 07:07:11 浏览: 39
CSMA/CA协议是一种用于无线网络中的MAC协议,主要用于解决无线介质带来的冲突问题。下面是一个简单的C++实现。
首先,我们需要定义一个结构体来表示每个节点:
```c++
struct Node {
int id; // 节点ID
bool busy = false; // 是否繁忙
bool sent = false; // 是否发送了数据包
bool channel_busy = false; // 信道是否繁忙
int backoff = 0; // 后退指数
int cw = 4; // 竞争窗口大小
int cw_max = 1024; // 最大竞争窗口大小
int timeout = 16; // 超时时间
int rts_timeout = 4; // RTS超时时间
int cts_timeout = 4; // CTS超时时间
};
```
然后我们需要定义一些常量来表示一些参数:
```c++
const int NODE_NUM = 5; // 节点数量
const int SEND_NUM = 3; // 发送数据包数量
const int RTS_NUM = 2; // 发送RTS包数量
const int CTS_NUM = 2; // 发送CTS包数量
const int MAX_ROUND = 10; // 最大轮数
const int PACKET_SIZE = 1000; // 数据包大小
```
接下来我们实现一些辅助函数:
```c++
int rand_between(int a, int b) { // 随机生成a到b之间的整数
return rand() % (b - a + 1) + a;
}
void backoff(Node& node) { // 后退指数减一,如果为0则重传
if (node.backoff == 0) {
node.cw = min(node.cw * 2, node.cw_max);
node.sent = false;
} else {
node.backoff--;
}
}
int channel_busy_count(vector<Node>& nodes) { // 统计信道繁忙的节点数量
int count = 0;
for (auto& node : nodes) {
if (node.channel_busy) {
count++;
}
}
return count;
}
void send_rts(Node& node, vector<Node>& nodes) { // 发送RTS包
node.busy = true;
node.sent = true;
node.channel_busy = true;
node.backoff = rand_between(0, node.cw - 1);
for (auto& n : nodes) {
if (n.id != node.id) {
n.channel_busy = true;
}
}
}
void send_cts(Node& node, vector<Node>& nodes) { // 发送CTS包
node.busy = true;
node.sent = true;
node.channel_busy = true;
node.backoff = rand_between(0, node.cw - 1);
for (auto& n : nodes) {
if (n.id != node.id) {
n.channel_busy = true;
}
}
}
void send_packet(Node& node, vector<Node>& nodes) { // 发送数据包
node.busy = true;
node.sent = true;
node.channel_busy = true;
node.backoff = rand_between(0, node.cw - 1);
for (auto& n : nodes) {
if (n.id != node.id) {
n.channel_busy = true;
}
}
}
void handle_packet(Node& node, vector<Node>& nodes) { // 处理数据包
node.busy = false;
node.channel_busy = false;
node.backoff = 0;
node.cw = 4;
node.sent = false;
}
```
最后我们实现主函数:
```c++
int main() {
srand(time(0)); // 初始化随机种子
vector<Node> nodes(NODE_NUM); // 创建节点
int round = 1;
while (round <= MAX_ROUND) { // 最多进行MAX_ROUND轮
cout << "Round " << round << endl;
for (auto& node : nodes) { // 每个节点处理一次
if (node.busy) { // 如果节点繁忙,则后退指数减一
backoff(node);
} else if (!node.sent) { // 如果节点没有发送过数据包
if (channel_busy_count(nodes) == 0) { // 如果信道空闲
if (rand() % 2 == 0) { // 有50%的概率发送数据包
send_packet(node, nodes);
cout << "Node " << node.id << " sends a packet." << endl;
} else { // 有50%的概率发送RTS包
send_rts(node, nodes);
cout << "Node " << node.id << " sends an RTS packet." << endl;
}
} else { // 如果信道繁忙,则后退指数减一
backoff(node);
}
} else if (node.sent) { // 如果节点发送过数据包
if (node.timeout == 0) { // 如果超时,则后退指数减一
backoff(node);
cout << "Node " << node.id << " times out." << endl;
} else {
node.timeout--;
}
}
}
for (auto& node : nodes) { // 处理RTS包和CTS包
if (node.sent && node.timeout == 0) { // 如果RTS或CTS超时
if (node.rts_timeout > 0) { // 如果是RTS超时,则重新发送RTS包
node.rts_timeout--;
send_rts(node, nodes);
cout << "Node " << node.id << " re-sends an RTS packet." << endl;
} else if (node.cts_timeout > 0) { // 如果是CTS超时,则重新发送CTS包
node.cts_timeout--;
send_cts(node, nodes);
cout << "Node " << node.id << " re-sends a CTS packet." << endl;
} else { // 如果RTS和CTS都超时,则后退指数减一
backoff(node);
cout << "Node " << node.id << " times out." << endl;
}
}
if (node.busy && node.sent && node.cts_timeout == 0) { // 如果CTS超时
backoff(node);
cout << "Node " << node.id << " times out." << endl;
}
}
for (auto& node : nodes) { // 处理收到的数据包
if (node.channel_busy) { // 如果信道繁忙
if (node.sent && node.rts_timeout > 0 && node.cts_timeout > 0) { // 如果节点发送过RTS包和CTS包
cout << "Node " << node.id << " receives a packet." << endl;
handle_packet(node, nodes);
} else { // 如果节点没有发送过RTS包和CTS包,则忽略数据包
cout << "Node " << node.id << " ignores a packet." << endl;
}
}
}
round++;
}
return 0;
}
```
这样我们就实现了一个简单的CSMA/CA协议的C++程序。注意这只是一个简单的实现,实际中可能需要根据具体情况进行一些修改。
相关推荐
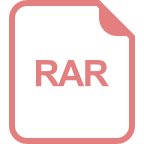








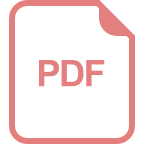
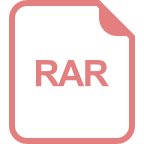