ds18b20温度传感器stm32读写数据代码
时间: 2023-08-14 16:04:35 浏览: 234
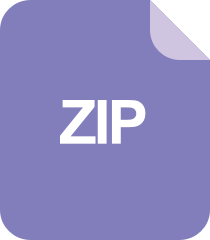
STM32单片机读写DS18B20温度传感器例程软件例程源码.zip

以下是DS18B20温度传感器在STM32上读取温度的代码示例:
```c
#include "stm32f4xx.h"
#include "delay.h"
#define DQ_OUT(x) if(x)GPIOB->BSRR=1<<5;else GPIOB->BSRR=1<<21;
#define DQ_IN GPIOB->IDR&GPIO_IDR_IDR_5
// DS18B20指令
#define DS18B20_SKIP_ROM 0xCC // 跳过ROM,忽略地址
#define DS18B20_CONVERT_T 0x44 // 启动温度转换
#define DS18B20_READ_SCRATCHPAD 0xBE // 读取暂存器
#define DS18B20_WRITE_SCRATCHPAD 0x4E // 写暂存器
void DS18B20_Reset(void)
{
DQ_OUT(0); // 拉低总线
delay_us(500); // 延时500us
DQ_OUT(1); // 释放总线
delay_us(30); // 延时30us
}
void DS18B20_WriteByte(uint8_t dat)
{
uint8_t i;
for(i=0;i<8;i++)
{
DQ_OUT(0); // 拉低总线
delay_us(2); // 延时2us
if(dat&0x01)DQ_OUT(1);// 写1
else DQ_OUT(0); // 写0
dat>>=1; // 右移一位
delay_us(60); // 延时60us
DQ_OUT(1); // 释放总线
delay_us(2); // 延时2us
}
}
uint8_t DS18B20_ReadByte(void)
{
uint8_t dat=0,i;
for(i=0;i<8;i++)
{
dat>>=1;
DQ_OUT(0); // 拉低总线
delay_us(2); // 延时2us
DQ_OUT(1); // 释放总线
delay_us(5); // 延时5us
if(DQ_IN)dat|=0x80; // 读数据
else dat&=~0x80;
delay_us(53); // 延时53us
}
return dat;
}
/**
* @brief DS18B20温度转换
* @param None
* @retval None
*/
void DS18B20_ConvertTemp(void)
{
DS18B20_Reset(); // 复位
DS18B20_WriteByte(DS18B20_SKIP_ROM); // 跳过ROM
DS18B20_WriteByte(DS18B20_CONVERT_T); // 启动转换
}
/**
* @brief DS18B20读取温度
* @param None
* @retval 温度值
*/
float DS18B20_ReadTemp(void)
{
uint8_t tempL,tempH;
int16_t temp;
float t;
DS18B20_Reset(); // 复位
DS18B20_WriteByte(DS18B20_SKIP_ROM); // 跳过ROM
DS18B20_WriteByte(DS18B20_READ_SCRATCHPAD); // 读暂存器
tempL=DS18B20_ReadByte(); // 读温度低8位
tempH=DS18B20_ReadByte(); // 读温度高8位
temp=((tempH<<8)|tempL); // 合成温度值
t=temp*0.0625; // 转换为实际温度值
return t;
}
int main(void)
{
float temp;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB,ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin=GPIO_Pin_5;
GPIO_InitStructure.GPIO_Mode=GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType=GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed=GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_PuPd=GPIO_PuPd_UP;
GPIO_Init(GPIOB,&GPIO_InitStructure);
while(1)
{
DS18B20_ConvertTemp(); // 温度转换
delay_ms(1000); // 等待1秒
temp=DS18B20_ReadTemp(); // 读取温度
}
}
```
注意:此代码仅供参考,具体实现需要依据开发板和传感器的不同进行修改。
阅读全文
相关推荐
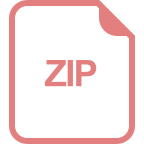
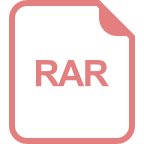
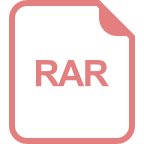
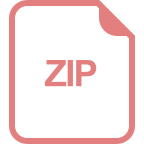
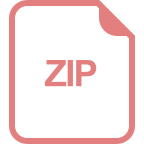
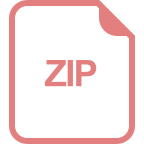
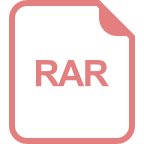
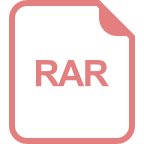
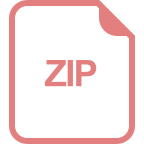
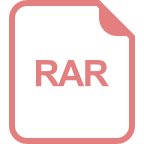
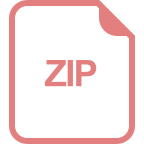
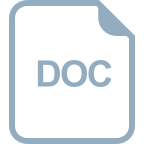
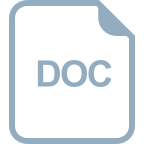
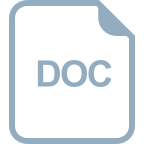
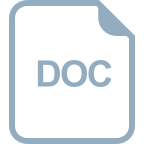
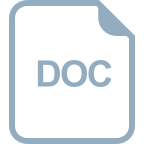