用cpp代码写一个注册案例
时间: 2023-05-30 08:01:56 浏览: 218
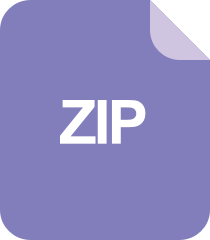
cpp-example
以下是一个简单的注册案例,使用了文件存储用户信息:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
// 用户信息结构体
struct UserInfo {
string username;
string password;
int age;
};
// 存储用户信息的文件路径
const string FILE_PATH = "user_info.txt";
// 读取所有用户信息
vector<UserInfo> readAllUsers() {
vector<UserInfo> users;
ifstream fin(FILE_PATH);
string line;
while (getline(fin, line)) {
UserInfo user;
int pos = line.find(',');
user.username = line.substr(0, pos);
line = line.substr(pos + 1);
pos = line.find(',');
user.password = line.substr(0, pos);
line = line.substr(pos + 1);
user.age = stoi(line);
users.push_back(user);
}
fin.close();
return users;
}
// 添加新用户
void addUser(const UserInfo& user) {
ofstream fout(FILE_PATH, ios::app);
fout << user.username << "," << user.password << "," << user.age << endl;
fout.close();
}
// 检查用户名是否已存在
bool isUsernameExists(const string& username) {
vector<UserInfo> users = readAllUsers();
for (const auto& user : users) {
if (user.username == username) {
return true;
}
}
return false;
}
// 注册新用户
void registerUser() {
string username, password;
int age;
// 获取用户输入
cout << "请输入用户名:";
cin >> username;
if (isUsernameExists(username)) {
cout << "该用户名已存在,请重新输入!" << endl;
return;
}
cout << "请输入密码:";
cin >> password;
cout << "请输入年龄:";
cin >> age;
// 创建新用户并保存
UserInfo user = {username, password, age};
addUser(user);
cout << "注册成功!" << endl;
}
int main() {
registerUser();
return 0;
}
```
阅读全文
相关推荐
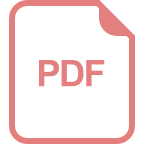
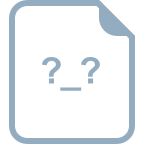
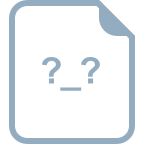
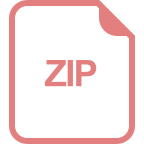
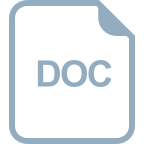
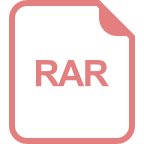
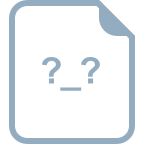
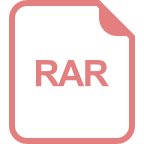
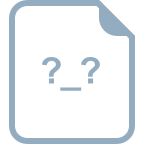
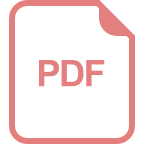
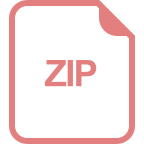
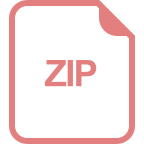
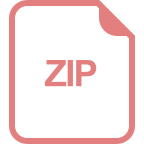
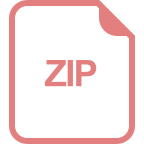
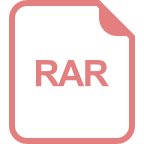
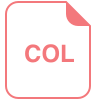
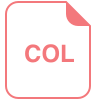