用C++写一个可以在Visual Studio上运行的排课程序
时间: 2023-12-04 18:03:22 浏览: 105
好的,下面是一个简单的排课程序的代码实现,你可以在Visual Studio中创建一个新的C++控制台项目,将代码复制粘贴进去,然后编译运行。
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
#include <fstream>
#include <sstream>
using namespace std;
struct Course {
string name;
int credits;
vector<int> conflicts; // 与之冲突的课程编号
};
vector<Course> courses; // 所有课程
vector<vector<int>> schedule; // 课程表
bool has_conflict(int course_num, int time_slot) {
// 判断某个课程在某个时间是否与已安排的课程冲突
for (int i = 0; i < schedule[time_slot].size(); i++) {
int other_course_num = schedule[time_slot][i];
if (find(courses[course_num].conflicts.begin(), courses[course_num].conflicts.end(), other_course_num) != courses[course_num].conflicts.end()) {
return true;
}
}
return false;
}
int get_credit(int time_slot) {
// 统计某个时间段的总学分数
int credit = 0;
for (int i = 0; i < schedule[time_slot].size(); i++) {
int course_num = schedule[time_slot][i];
credit += courses[course_num].credits;
}
return credit;
}
void print_schedule() {
// 打印课程表
cout << "Course Schedule:" << endl;
for (int i = 0; i < schedule.size(); i++) {
cout << "Time Slot " << i + 1 << ": ";
if (schedule[i].size() == 0) {
cout << "No class scheduled" << endl;
}
else {
for (int j = 0; j < schedule[i].size(); j++) {
int course_num = schedule[i][j];
cout << courses[course_num].name << " ";
}
cout << "(" << get_credit(i) << " credits)" << endl;
}
}
}
int main() {
// 读入课程信息
ifstream infile("courses.txt");
string line;
while (getline(infile, line)) {
Course course;
istringstream iss(line);
iss >> course.name >> course.credits;
int conflict_num;
while (iss >> conflict_num) {
course.conflicts.push_back(conflict_num);
}
courses.push_back(course);
}
// 初始化课程表
int num_time_slots;
cout << "Enter the number of time slots: ";
cin >> num_time_slots;
for (int i = 0; i < num_time_slots; i++) {
vector<int> time_slot;
schedule.push_back(time_slot);
}
// 排课
for (int i = 0; i < courses.size(); i++) {
bool scheduled = false;
for (int j = 0; j < num_time_slots && !scheduled; j++) {
if (!has_conflict(i, j) && get_credit(j) + courses[i].credits <= 24) {
schedule[j].push_back(i);
scheduled = true;
}
}
if (!scheduled) {
cout << "Error: Could not schedule " << courses[i].name << endl;
}
}
// 打印课程表
print_schedule();
return 0;
}
```
这个程序读入一个文件 `courses.txt`,文件格式如下:
```
Course1 3 2 3 4
Course2 4 1 3
Course3 2 1 2
Course4 3 2 4
```
其中每一行表示一门课程,第一个字段是课程名称,第二个字段是学分数,后面的字段是与之冲突的课程编号。程序会根据课程信息和时间段的数量,自动排出一个课程表并打印出来。
阅读全文
相关推荐
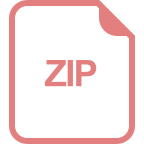
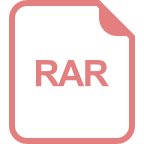
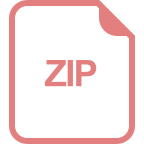
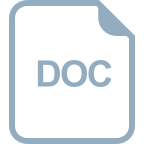
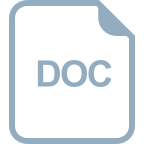
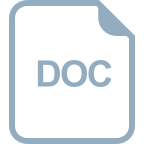
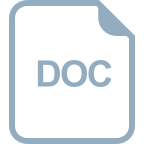
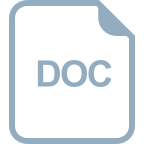
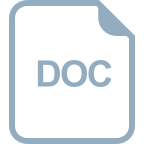
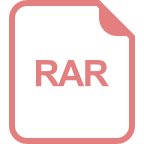
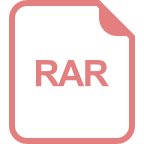
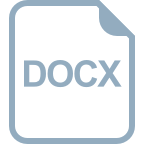