用c语言编写输入一个字符串,统计数字字符的个数
时间: 2024-05-13 18:14:12 浏览: 145
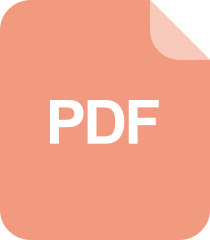
基于C语言的字数统计

下面是一个简单的 C 语言程序,用于输入一个字符串并统计其中的数字字符个数:
```c
#include <stdio.h>
#include <ctype.h>
int main()
{
char str[100];
int i, count = 0;
printf("请输入一个字符串:");
scanf("%s", str);
for (i = 0; str[i] != '\0'; i++) {
if (isdigit(str[i])) {
count++;
}
}
printf("数字字符的个数为:%d\n", count);
return 0;
}
```
该程序首先使用 `scanf()` 函数获取用户输入的字符串,然后使用 `isdigit()` 函数判断每个字符是否为数字字符(即 `'0'` 到 `'9'` 之间的字符),最后通过一个计数器变量统计数字字符的个数并输出结果。
阅读全文
相关推荐















